Getting Started
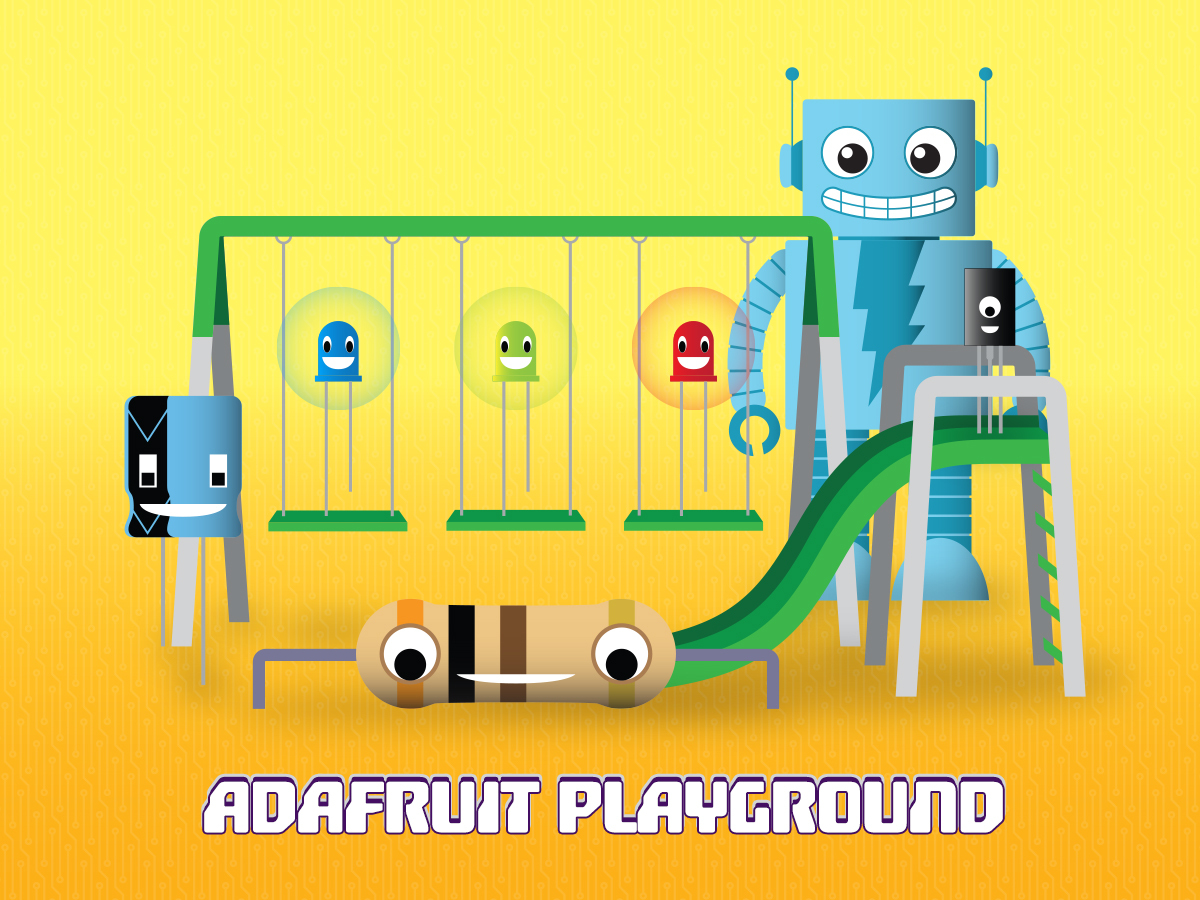
Adafruit Playground is a wonderful and safe place to share your interests with Adafruit's vibrant community of makers and doers. Have a cool project you are working on? Have a bit of code that you think others will find useful? Want to show off your electronics workbench? You have come to the right place.
The goal of Adafruit Playground is to make it as simple as possible to share your work. On the Adafruit Playground users can create Notes. A note is a single-page space where you can document your topic using Adafruit's easy-to-use editor. Notes are like Guides on the Adafruit Learning System but guides are high-fidelity content curated and maintained by Adafuit. Notes are whatever you want them to be. Have fun and be kind.
Click here to learn more about Adafruit Playground and how to get started.
-
ESPTOOL-JS with Partition Table listing + Data saving - Easily backup your device before installing circuitpython/whatever I'm often wanting to list what's on a device in terms of partition table. There's a handy utility included with the ESP-IDF, but it's python only and it only accepts files so you have to first call esptool to save your partition table data. I've had to do it so often that I've saved the two commands in another playground note, but not any longer!
The other thing is I swear that there used to be some online place to download your devices flash for backup using web serial, but try as I might I cant find such a place, and my google-fu is usually on point - ignoring my switch to duckduckgo...I digress, introducing my latest possibly needless project, esptool-js "partition edition":
How to use it?
Well, the image above shows after I've clicked the Read Partition Table button. The default partition offset of 0x8000 should be fine for most boards, otherwise try 0x9000, although custom partition table offsets are supported. You won't even see those options until you've clicked Connect and selected your boards serial port.
Once your partition table has loaded it shows the expected information, the bit most people are usually interested in is the first app partition, or ota_0 in this case, and the user file system (user_fs), which is a FAT partition in this screenshot.
The final column in the table has the download button for each partition, clicking it will start reading from the flash memory of the connected device, and once finished will ask the browser to download it. It will be named according to the chip name, partition type, starting offset, and size in bytes, but all the numbers are in hex not decimal.
-
Tiny Terminal with a Magnetic Connector Those nice magnetic connectors (four pins) were waiting to be used in a project, until now.
I needed a way to peek in on a UART serial stream, but did not want to have a full laptop along for the ride. So I made what is not that different from those old DEC VT-200 terminals.
An RP-2040 to listen to the UART and send the text to the tiny Monochrome 0.96" 128x64 OLED Graphic Display.
The Circuitpython code is simple with a just a bit work to get the all the previous line of the terminal display to move up.
So I use of this for a project that logs data, sent out a UART (as well as to an SD card). Wire the UART to a mag connector, in my case I put Power and Ground on either side while the TX and RX pins are in the middle, but there's not really a standard (until now ;).
Here is code for the monitorand then it pretty easy to add code to any logging project to (also) send Serial Data out on a UART TX pin. I also wired up (optional) 5 volt power and (not optional) ground.
TBD add fritz diagrams, images and more code... -
Adding WIFI the Easy Way Adding WIFI the Easy Way
If you start from scratch, the best thing is to buy a board with integrated WIFI. But this is not possible in all cases. Many older boards just don't have a suitable chip. This is especially true for boards that are based on the RP2040.
For example I recently bought the TinyFX from Pimoroni. That is a very small board about the size of a Qt-Py for controlling Lego lighting kits:
Another example is the Tufty2040, a large sized display with a RP2040 on board:
But I have also quite a few other boards lying around that would profit from connectivity e.g. the ItsyBitsy M4 Express. And of course I also own some of the shiny new Pico2 boards.
Airlift
The standard solution for adding WIFI is Airlift. Airlift is a WIFI-coprocessor running a special firmware on an Espressif ESP32-WROOM-32. Besides the "pure" chip on a breakout, there are also addons for specific form-factors like a FeatherWing or a BitsyWing.
But Airlift has two major problems. The first is wiring. Airlift is based on SPI and in addition uses a number of control GPIOs. So if you don't use one of the wings (that block all of your pins) you end up with wiring 7-9 jumper-cables. That is not only cumbersome, but the boards above don't even provide that many pins (the TinyFx e.g. just exposes what you see in the image).
The second problem is software. The Airlift uses a special CircuitPython library that does it's job, but in a slightly different way than the standard WIFI support of CircuitPython does. E.g. instead of connecting with
wifi.radio.connect()
you useesp.connect_AP()
and instead of usingwifi.radio.connected
you check the connection state withesp.is_connected
. There are many other differences in the API. So all the boilerplate code you have or download from the internet has to be adapted for the Airlift. As long as this is your maincode.py
file, this is no major problem. But once you start using libraries things get complicated. You have to install the source version of the libs and adapt them. Maintaining this is no fun.ESP-AT
An alternative to Airlift is ESP-AT. This is a firmware maintained and provided by Espressif turning many of their chips into WIFI-coprocessors. ESP-AT is highly customizable but in its standard versions it uses plain UART for the communication with the main MCU. So you basically only need four wires: VCC, GND, RX and TX.
The drawback of ESP-AT is its textual interface: you send so called "AT-commands" to the co-processor and then you have to parse the response to extract the relevant data. Some of these commands are really complicated, e.g. to connect to an AP you would send
AT+CWJAP="myssid","mypasswd",,0,1,3,1,5,1
Wrapping all the complexity in a library really makes sense. For this reason, Adafruit had created the library CircuitPythonESPAT_Control, but it was abandoned in favor of Airlift and is no longer maintained. That library also suffers from the same drawback as the Airlift library, i.e. it uses a different API than standard CircuitPython.
CircuitPython-ESP32AT
For this reason I created a new library called CircuitPython-ESP32AT. This library talks to the ESP-AT on an ESP-coprocessor, but instead of inventing yet another API it uses the standard CircuitPython API for
wifi
,ssl
,socketpool
and so on.At first, this might seem confusing. On boards with native WIFI these modules don't need to be installed, since the CircuitPython firmware already provides them. For other boards, the ESP32AT project now provides drop-in modules with the same names providing (almost) the same set of functionality.
One important function the external modules don't provide is the web-workflow which really needs native WIFI. Otherwise, you need to look hard to spot differences.
You will find installation and setup instructions as well as notes about various hardware alternatives in the repo of the library. Instead of reproducing this information here, I only show some examples I actually use.
Hardware
From hardware side, you need a board with a supported ESP-chip. Any ESP32, ESP32-S2 and ESP32-Cx will do. ESP32-S3 and the ESP32-Hx series are not supported. You can even use very old ESP8266 hardware as long as it has 1MB of flash. But this is not really recommended since the ESP8266 only supports operation in TCP/UDP/SSL client mode. In addition, you have to compile the firmware yourself (which in fact is easy and a matter of a quarter of an hour - see the repo for details).
Wiring is easy: you must connect RX/TX of your host MCU to the AT-UART ports TX/RX of the coprocessor. Besides RX/TX you should also connect GND. Powering the coprocessor directly from the host MCU might or might not work. For example, I had no problems powering the ESP32C3-SuperMini from the 3V3 pin of the Pico/Pico2, but I did not use any other peripherals:
The connection in this image uses a trick: it redefines the I2C-pins of the PiCowbell adapter from I2C to UART. Of course you loose I2C on these pins, so this is not a solution for all problems but it demonstrates how simple wiring can be compared to the Airlift.
I am using the same trick for the TinyFX board from above. The board does not have any dedicated UART-pins, but the pins of the Stemma/Qt can also be repurposed to UART instead of I2C. This allows a very simple connection using a Stemma/Qt-DuPont adapter cable for the connection of the TinyFX to again to an ESP32C3:
Even simpler is the usage together with the Maker Pi Pico Board. This board has a socket designed for the ESP-01S:
Liligo has created a chip with the same form-factor and basically the same pinout, which is called Lilygo T-01 C3. This board works also in the socket and gives full performance and provides all WIFI-functionality.
The Swedish company "iLabs" even has a Feather-sized RP2350 board with an integrated ESP32C6:
Ok, we will see a Pico2-W soon, but until then, this is the best solution for a WIFI-enabled RP2350.
Performance
For most use-cases like updating the time, querying an API (e.g. for weather information) or running an AP with a webserver the performance is just on par with native WIFI. There are some special cases though where native WIFI is better: e.g. I have a high-frequency data-sampling application that sends data using UDP to a reciever. Native WIFI can send with a rate of 0.001s per message, using ESP-AT this is much slower (I measured 0.015s per message).
Also, if you are dealing with large responses the default chunk size of 32 bytes that the
adafruit_requests
library uses will slow you down, since every chunk is an AT-transaction. Usingresponse.iter_content()
directly is a workaround to speed up processing (this also helps native WIFI a bit and Airlift too). -
I turned the Raspberry Pi 400 into a Fallout-style terminal! I'm almost ashamed to admit I had a Raspberry Pi 400 that went unappreciated for the longest time. My Dad got it for me as a gift, and it was sitting in a box of spare parts for months. Once I finally got to open it up and take a good look at it, I really wanted to do something cool with it. The Pi 400 was great enough by itself, having a built-in keyboard which you would only need to add a monitor to have a complete computer setup. However, I wanted to have an "all-in-one" setup, with even less wires to plug in. And since I was in a "Fallout" kinda mood, having recently watched the TV series and played one of their games, I decided to model this all-in-one casing based off of the terminal featured in the Fallout game series.
The general idea was to build a plastic casing to house all the pieces, and extend the Pi 400's ports with panel mount cables. First things first, I needed a monitor. It took me a while to find a monitor that I liked, but in the end, the one pictured above was what I settled on. However, I realized much too late that the monitor in question turned out to be a 12V monitor, and I was trying to have all the pieces be consistently 5V. I needed to adapt my strategy if I wanted to have a system powered by only one adapter. So, I bought a power adapter that output 12V and 5A, which I would use to power the monitor first, then bring the remaining power down to 5V via a buck converter.
-
Guide: Zapper Lights/Sound mod If you grew up with a Nintendo Entertainment System, you probably remember playing games like Duck Hunt that used the Nintendo Zapper "light gun". The Zapper used a clever technique that depended on the game being displayed on a Cathode Ray Tube, or CRT television. These days, CRTs are rare, and are often prized collectors items among retro gaming enthusiasts. Since these Zappers don't work on modern TVs, most people don't have much use for them anymore. So, why not convert it into a toy/prop that lights up and plays sounds?
Overview
This project uses a Prop-Maker Feather RP2040 to repurpose the the Zapper's iconic shell and clicky trigger mechanism into a prop/toy that uses a NeoPixel for a "muzzle flash", a speaker to play sound effects, and 3D printed parts to contain the electronics. The code is written in CircuitPython, and sounds can be changed or added by dragging and dropping files with no code changes required!
-
PC media remote Program your device
Installing CircuitPython environment
- Download .uf2 file here:
- Plug in USB cable from PC to RP2040 board while holding the BOOTSEL button.
- A new drive should get mounted on your system.
- Drag/drop downloaded .uf2 file onto newly mounted drive (RPI-RP2).
Installing libraries and project code
From "CircuitPython Library Bundle" (download here):
- Copy from bundle .zip file to the microcontroller [drive:]\lib\ folder:
adafruit_hid
From the PCMediaRemote release page (download here):
- Download and unzip the "Source code (zip)" file from the "Assets" section.
- Copy the "unzipped" custom project code to microcontroller [drive:]:
- [PCMediaRemote folder]\lib_cktpy\* => [drive:]\lib\
- [PCMediaRemote folder]\pkg_install\MediaRemote_RP2040\*.py => [drive:]\
NOTE: Feel free to check for newer versions of PCMediaRemote on the releases page. Code from the main branch might also be functional, but explicit releases are less likely to have issues.
Customizing your solution
You can directly modify the main.py file on the [drive:]\ folder. Every time you save the file, CircuitPython will restart with the updates applied.
Note that if the file gets corrupted for some reason, your changes will be lost. It is recommended to work from a folder on your PC, and manually upload (ex: drag/drop) the changes to the CircuitPython drive.
Overview
A media remote receiver for your PC/MAC/thing supporting keyboard media keys.
- Also works with many smart TVs and phones.
- Flexible CircuitPython-based solution can easily be adapted to other microcontrollers/IR remotes.
- Built-in IR signal decoder utility on serial monitor output.
- An easy, inexpensive, solderless build! (if desired)
-
TM1814 LEDs with CircuitPython & RP2040 There are a lot of addressable LEDs out there. The chief variant is NeoPixel .. NeoPixel and Dotstar. Our two types of addressable LEDs are NeoPixel and Dotstar .. and TM1814....
(you get it right? It's like the Spanish Inquisition skit, but about LEDs?)
Long story short: TM1814 addressable LEDs can work with CircuitPython on the RP2040, but you'll have to use some custom code to talk to them. The TM1814PixelBackground class is a PixelBuf, though, so you can use it with the LED animation library no problem.
There's one big difference with TM1814 LEDs: The logic levels are inverted compared to NeoPixels. And, the first 64 bits are an overall brightness value. Two differences. The bits are inverted, and the timing is subtly different, and there's an overall brightness value. And if you don't continuously update the pixels, they enter a test mode. Four. Four main differences. And often the strips are powered from a higher voltage like 12V.
You have one last chance left to show me the source code
But first! You'll want to make sure you're using CircuitPython 9.2, at least 9.2.0-beta.0 or newer. It seems there was a teensy, tiny bug in CircuitPython that you'd encounter if you used this code. So with that out of the way
Two, I'll allow you two last chances to show me the source code
Grab the code from below (or from github) and place it on your CircuitPython device as code.py. Then, hook up your TM1814 strip to CircuitPython's GND and A0 pins. Hook up appropriate power to the TM1814 strip (mine was labeled "12V", so I used a 12V supply. 5V definitely didn't work! And definitely don't cross-connect the LED strip's supply into the RP2040's VCC or +5V!).
When the CircuitPython LED code is not running (or if it malfunctions) you get an obnoxious test pattern generated by the TM1814 LEDs themselves. Sorry, there's nothing we can do about it.
Once the code's running you'll get a comparatively soothing rainbow LED animation instead.
If you won't show us the source code I'm afraid we'll have to put you in the Comfy Chair
-
Make Code on the Go - Makecode.Adafruit, that is.... I am a big fan of "being able to code whenever/wherever I am." That's why I bought a wireless keyboard for my iPhone, when I realized I could edit CircuitPython programs using my phone. It's one of my favorite things about the Micro:Bit - the App lets you write and upload code from your phone.
But I didn't think I could do that with makecode.adafruit.com programs for the Circuit Playground. I was wrong, in fact it's pretty easy. I just needed a lightning-to-USB adapter so I could download the code!
First - load your code in your browser (like the picture above).
Next - click the download icon in the lower left.
Then, click the file link above, to "open in a new tab."
-
A PyDOS Handheld I was looking to try out my new Adafruit Feather RP2350 and I remembered I had the Solder Party Keyboard Featherwing.
The Keyboard Featherwing is a handy device: it is about the same size as a BlackBerry phone with the same alphanumeric keyboard and a 2.6" 320x240 color display. Plug in a Feather board as the "brains" and you have a portable system.
Alas, one has to program the Feather, preferably with CircuitPython, to use the keyboard, display, and other features. Solder Party has example code snippets for those features. But what about something more holistic, more like a computer with input and output?
I found two solutions that were perfect: PyDOS and Beryllium OS. Both are built on top of CircuitPython.
PyDOS emulates MS-DOS commands used on PC compatible computers. And Beryllium OS, formerly ljinux, acts as a Linux-like computer. Neither are binary compatible (they cannot run native DOS or Linux binaries) but their commands and interactions emulate those operating systems.
This Playground Note will show you how I built my PyDOS handheld in short order.
There are two videos, one from Adafruit Show and Tell and another for Tom's Hardware The PiCast.
Preparing the Feather
Solder male pin headers onto the Feather RP2350. You could use long pin stacking headers to add a FeatherWing, but that would create quite a stack on the back.
See this guide page for soldering details:
-
Home Assistant WiFi Button with CircuitPython Have you ever wanted to connect a simple button or sensor to Home Assistant using Circuit Python? This script demonstrates registering a device with Home Assistant so that it can be automatically connected to an Automation. The script triggers its event on power-on. The example uses the "trigger" type, which is better than "button" for a simple remote control. Other MQTT integrations could put a sensor reading on the dashboard, or turn your CircuitPython board into a WiFi light or fan.
Ingredients
- ESP32-C3 board running CircuitPython 9.1.4
- Home Assistant with the MQTT integration and broker, accessible to the local network
This script also demonstrates setting a BSSID, a 12-digit hexadecimal string, to connect to a specific access point.
I was not able to connect to my local
mosquitto
MQTT broker over ssl; something about how the the MQTT client and broker interact prevents CircuitPython from validating the certificate. The comments include an example of loading a custom root certificate for the connection.How It Works
Home Assistant's MQTT integration listens on the
homeassistant/device_automation/#
topic for discovery messages. When it sees a properly formatted message, it adds or updates that device underSettings > Devices and Services > MQTT > devices
. It also listens ontopic
, herecircuitpy/{unique_id}/action
, for commands from the device. For a two-way device, you can also set a topic for Home Assistant to send commands back to CircuitPython.Now, we can add our device to an automation in Home Assistant without any additional configuration.
Going Further
Can we send our configuration messages with
mqtt_client.publish(topic, msg, retain=True)
so Home Assistant will remember us, even if it restarts before we do?Can we configure our CircuitPython board as a Home Assistant temperature sensor?
Can we put the device in low power mode, wake on a pin change, and make a real battery-operated remote control?
Use nginx to proxy SSL instead of mosqitto's idiosyncratic support.
See also
-
Project Nyota Language tools for NeoTrinkey
Inspired by Star Trek's Nyota Uhura, these programs provide a way to use a NeoTrinkey to review alien (or foreign) language words or phrases. They are all contained in my github archive Project Nyota.
There are two programs, langtutor.py and langtest.py - copy the one you want to use to code.py. The helper files wise.py and prt.py are required.
The file "langs" is a list of the languages to review. Each line in "langs" should be the name of a file containing language information. In this archive the languages are: klingon, vulcan, mandoa, and Swahili (in honor of Uhura - "Nyota" means "star" in Swahili).
Language files should be in the form:
"Word-or-phrase", "target-language-translation" "Word-or-phrase", "target-language-translation" "Word-or-phrase", "target-language-translation" ....
When either program runs, you'll see:
number of languages: 4 klingon vulcan mandoa swahili Current lang: klingon
To toggle between languages, touch pad #1. When you reach the one you want to review (for the langtutor.py program) touch pad #2 and you'll see 5 random review pairs. For example from the Mando'a set:
You're right. : Gar serim. twenty : ad'eta seventy : tad'eta eighty : shehn'eta Good. : Jate.
With the langtest.py program, when you choose a language and touch pad#2, you'll get four tests where you're given a word or phrase, then a choice of two possible answers in the target language. Touch #1 or #2 to choose. A pixel will light green or red to indicate if you are right or wrong. (If wrong, the correct answer will be given). After four questions, you can touch #2 to get four more, or touch #1 to change languages.
For example:
Current lang: vulcan advise 1:lahso 2:a'Tha correct! walk (action-word) 1:imroy 2:lahso yes! 'logic', reality-truth, the way things are. 1:c'thia 2:lahso wrong: c'thia 'immanence' direc experience of the creator 1:kah-hir 2:a'Tha correct! touch #2 for another quiz, or #1 to change language.
When running the programs, you can set the variable REPL to "True" or "False" to direct the output. If REPL=True, all output is sent to the REPL. If it is False, output is directed as if typed using the HID interface. There is a delay when that is the case, to give you time to switch to an open editor window to receive the output.
Note:
Copy all these files to the neotrinkey: langtutor.py, langtest.py, wise.py, prt.py, langs, klingon, vulcan, mandoa, swahili. Then copy langtutor.py or langtest.py to code.py to run.
Language sources:
- Swahili vocab from: https://www.fluentin3months.com/swahili-words/
- Mando'a vocab from https://mandoa.org/ Note: the Mando'a language from Star Wars was developed originally by the author Karen Traviss for the Mandalorian people
- Klingon vocab from: https://kli.org and https://hol.kag.org
- Vulcan vocab from: https://tinyurl.com/VulcanArchive - archive.org of Marketa Zvelbil's original Vulcan work (note: In case archive.org is not available, I've copied the Dictionary and Lexicon to vulcdict.txt and vulcanlex.txt)
- To create your own language, you can use a tool like this: https://rollforfantasy.com/tools/language-generator.php
Nyota Uhura -
Display AIO+ Local Weather Conditions: MatrixWeather System The objective of this project is to replace the existing WeatherMatrix project's MatrixPortal M4 display with a newer version that uses weather data provided by Adafruit IO Plus (AIO+) instead of the openweathermap.org web API. The requirements include:
- Implement with CircuitPython for ease of development and prototyping.
- Duplicate the existing 64x32 LED matrix display layout including label colors and icons.
- Develop an architecture to support multiple autonomous displays and local data feed devices (new).
- Utilize a weather data source that accurately aggregates station information that closely matches local conditions.
- Weather data updated approximately 3 times per hour and is reliably available.
- Service subscription is free or reasonably priced.
- Include data elements for wind gusts and local workshop temperature (new).
- Display a progress bar to indicate data time since last update (new).
- If possible, use the existing MatrixPortal M4 hardware; upgrade to MatrixPortal S3 only if necessary.
Special Acknowledgements
Thank you to John Park for the initial weather display design concept (see Weather Display Matrix) that inspired this project. Also, the work of Trevor Beaton was instrumental in creating the updated display design with a clearer and simpler coding approach (see itsaSNAP Daily Weather Forecast Board).
Choose a Weather Observation Source
Three options for sourcing weather data were considered. Here are some pros and cons of each.
openweathermap.org:
- utilizes the existing or similar web API; minimal code changes will be needed
- weather station aggregation often doesn't always match local conditions
- data retrieval throttling is reasonable with a consistent service level
- free service was phased out; will eventually require paying for the service
weather.gov:
- a moderately well-documented web API; moderate code changes will be needed
- weather station data is not aggregated and closely matches local conditions
- single weather station data is not reliably available, sometime for hours at a stretch
- data retrieval throttling is currently reasonable, but without a guaranteed service level
- a free service
AIO+ Weather (Apple WeatherKit):
- moderately well-documented CircuitPython library approach; major code changes will be needed
- weather station data is aggregated and matches local and iPhone conditions
- data retrieval throttling is reasonable with a consistent service level
- weather data and workshop conditions can be combined and viewed on a web-based IO dashboard
- requires a paid subscription to AIO+ for access to its Weather Service
The choice to use AIO+ Weather was an easy one since my existing weather tracking and corrosion monitoring projects require a subscription to AIO+. We'll need a new system design and a major rewrite of the existing MatrixWeather project code to make this happen, providing ample opportunities to improve performance and reliability. The next step was to redesign the overarching weather system architecture.
See weather.gov: A Truly Free Weather API and AIO+ Weather: A Premium Alternative for Local Observations for a detailed discussion of each alternative.
-
Installing Python 3.13 using uv I use Debian Linux; the current stable version of Debian has Python 3.11.2. At the time I write this, Python 3.13 has just been released. So I used my desire to try out the new Python as a chance to explore uv as well. My instructions here are tested on Debian Linux, but may work with modifications on other Linux-based systems like Ubuntu or Raspberry Pi OS.
What's uv, anyway?
uv is "An extremely fast Python package and project manager, written in Rust." It's on github and pypi and is also well-documented.
Among its many features, uv is capable of managing multiple versions of Python, so I decided to use it to test drive Python 3.13.
Installing uv via pipx
First, install the pipx command using the operating system package manager. (debian package name: pipx)
Then, install uv:
pipx install uv
Once you've done this, you should have the
uv
command available. However, you may see a warning like this: -
Pumpkins vs Skeletons Game for CircuitPython This is a game about skeletons, pumpkins, and a catapult having a Spooky experience under the full moon. If you've thought about making a game in CircuitPython but aren't sure where to start, this project might be a useful source of ideas.
Charging a Pumpkin
This is how it looks when you hold the USB gamepad's A button to charge up a pumpkin.
-
Keyboard Featherwing Messenger powered by LVGL Several years ago Solder Party released the Keyboard Featherwing, a PCB that combines a Blackberry keyboard, a 320x240 TFT resistive touchscreen display, a 5-input DPAD, 4 tactile buttons, a microSD card reader, and more, all driven by an Adafruit Feather board of your choice. Eventually these were discontinued, I'm assuming because of the difficulty of sourcing the increasingly rare Blackberry keyboards. I didn't know what I wanted to do with them at the time, but I knew I was gonna want to do something with them at some point, so I ordered several of them before the stock was depleted.
One popular use for the Keyboard Featherwing has been to pair them with LoRa radio transceivers, to create a set of "Doomsday Messengers". These are devices that are able to send short text messages to each other using radio signals, sort of like walkie-talkies, but for text messaging. This makes sense: with the tactile keyboard, huge display, and instant compatibility with the Feather ecosystem (including the ability to use rechargable LiPo batteries), the Keyboard Featherwing practically seems designed for the use case!
I hacked together a quick demo a few years ago allowing for very basic communication between the devices, and then promptly lost interest. I wanted to write firmware that allowed for robust, reliable communication between the devices, but I also wanted something offering some of the affordances of a modern smartphone UI. If you've ever worked on UI for microcontrollers, you probably realize there are a lot of challenges. One of those challenges can be figuring out how to write a custom, complex UI with just the basic drawing functions provided by the commonly available drawing libraries. While possible, it can be really cumbersome once you start to want more modern UI features, such as widgets, scrolling, animation, multi-screen interfaces, and so on. Another challenge is implementing all of that in a performant way, given the limited speed and memory of most microcontrollers. At the time, I wasn't sure how I was to accomplish this without pulling my out my hair, so the project was put on the back burner.
Recently I decided to dig these up and give it another shot. I still wasn't sure exactly how I was going to do it, but I did have a concrete feature set in mind:
- The ability to send encrypted, reliable messages between two paired devices using LoRa technology
- The ability to pair each device with any other device using the same hardware and firmware, via a settings screen (i.e., no re-compilation needed to pair devices)
- The ability to modify and persist device settings and a small message history across power cycles
- Granular battery monitoring; specifically the ability to see the percentage of battery life remaining at any given time
- Heavy focus on physical controls, using the touchscreen to supplement the UI only where practical and/or necessary (if you aren't familiar with any of my previous projects, I'm a huge fan of physical controls)
Which Feather?