Getting Started
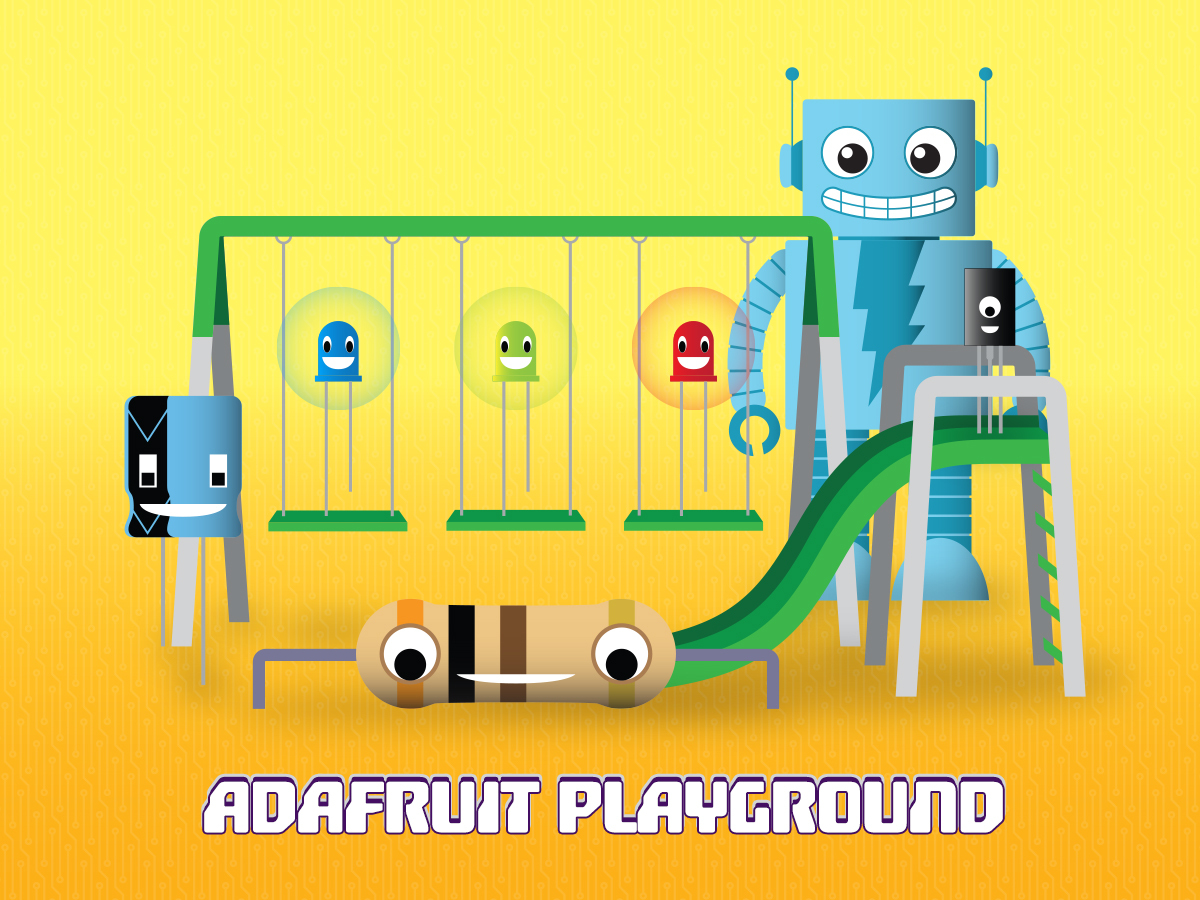
Adafruit Playground is a wonderful and safe place to share your interests with Adafruit's vibrant community of makers and doers. Have a cool project you are working on? Have a bit of code that you think others will find useful? Want to show off your electronics workbench? You have come to the right place.
The goal of Adafruit Playground is to make it as simple as possible to share your work. On the Adafruit Playground users can create Notes. A note is a single-page space where you can document your topic using Adafruit's easy-to-use editor. Notes are like Guides on the Adafruit Learning System but guides are high-fidelity content curated and maintained by Adafuit. Notes are whatever you want them to be. Have fun and be kind.
Click here to learn more about Adafruit Playground and how to get started.
-
E-Ink Countdown in CircuitPython with Custom Stand Along the way I learned a few things:
- How to take an Adafruit PCB design in Eagle format and import the board outline all the way into FreeCAD for locating dimensions and features
- How to make my code robust against transient errors like network errors
- How to make my code deep sleep for the right length of time
There are also a few things this code demonstrates that are less common knowledge:
- Using fonts from the font bundle
- Using the datetime module for arithmetic on dates & times
- Increasing reliability by re-trying operations that can fail
- Reducing battery usage by deep sleeping and avoiding connecting to WiFi
This project uses FreeCAD & KiCAD, both of which are Open Source software that are free to download & use.
Parts Needed
Code & Installation
I recommend using Adafruit circup to install the needed libraries for projects. Here's what you need to do:
- Install circup on your desktop computer
- Enable the "fonts bundle" by running this command (just once, circup remembers this setting):
circup bundle-add adafruit/circuitpython-fonts
- Copy code.py to your CIRCUITPY drive (Download it via the "raw" link at https://gist.github.com/jepler/b2c020a6caa65a31297053b7216fcc15)
- Run the following command to auto-install required libraries:
circup install -a
You'll also want to configure wifi on your device using settings.toml. For lower power usage, configure WIFI_SSID and WIFI_PASSWORD options. For web workflow but higher power usage, configure CIRCUITPY_WIFI_SSID and CIRCUITPY_WIFI_PASSWORD options.
-
Creating Reduced Sized Bitmap Fonts From .ttf File ... using FontForge
Because CircuitPython uses bitmapped fonts (.bdf) as well as the binary .pcf format but many free fonts online are in .ttf format, following the Custom Fonts for CircuitPython Displays learning guide I came up with a cheatsheet to quickly take a full font, select only the limited characters I wanted and generate a small .bdf file. As I only do it once in a while if I don't write it down I wouldn't remember. Below is all covered in the above linked learning guide, but I wanted a quick and dirty cheatsheet, so here it is.
-
Open .ttf font file in FontForge
-
Select characters wanted including space before the “!” (you may need to scroll up). This is important and is missed in the learning guide example screenshot. Without selecting the ASCII 32 spot before the "!" character you won't have a way to display a space, so your display willlooklikethis. See first graphic below showing red box of missing space character you want to be sure to select.
-
Besides the basics of numbers, letters, and punctuation, be sure to select any others you want. I have noticed many .bdf fonts, including CircuitPython's built in terminalio.FONT, don't include a degree symbol (e.g. 78°F will display as 78F) even though many projects people create include temperature. So grab that symbol plus others that might be useful such as ± as another example.
-
Edit -> Select -> invert selection (selects everything you don’t want).
-
Encoding -> Detach & remove glyphs… (everything you don't want is removed)
-
Element -> Simplify -> Simplify and click OK on the warning box.
-
Element -> Bitmap Strikes Available…
-
In 3rd field, Pixel Sizes, enter size you want to create. For example if you want a 36 pixel font then type "36" (the first two fields will auto fill with values). Note that when you create a .bdf file you are only creating a file for that one pixel size.
-
File -> Generate Fonts…. Pick location to save then click Generate. Accept default of Other = 96.
To create other sizes go to step 7 and change the pixel size to the next pixel size file you want to create from the same font and characters already selected earlier. For example if you had just created a 36 pixel font, now change it to 24 in step 8 and generate the new file (step 9). Repeat for as many sizes as you need.
Finally if you really want to make the files small, following the online instructions using the web based Adafruit tool you can convert the .bdf files you just created to even smaller .pcf files. As an example, a (downloaded from Adafruit but further reduced by me) Arial-Bold-36.bdf font which was already a very compact 25kB on my disk got reduced further to a Arial-Bold-36.pcf file of only 16kB.
-
-
Set your sensor polling frequency to anything - WipperSnapper + API "testing" Adafruit IO I work on Adafruit IO, and I've had this mischievous plan for a while to adjust my WipperSnapper no-code sensor devices to poll more frequently than the 30s minimum that the web interface offers, like maybe as low as 5seconds to avoid burning my toast (having recently tried measuring the particle emissions again but found it too infrequent).
So Saturday morning I was having a prod at the firmware (you can too as it's open source code) and watching the API calls in the network monitor to see where gets prodded when the user changes a sensor to read every 30s.
-
Launching from DS9!!! Anyone who has seen the miniature 3D printed Trek and Star Wars ships hanging over my workbench (thanks, Thingiverse!) won't be surprised how many of my projects take an SF bent. But I've also got a space station!
And that inspires this Circuit Playground Express project - launching probes into the Bajoran Worhmhole!
I've got a repository for the code at DS9-game:
There are three files, two CircuitPython, and one JavaScript (or you can follow the makecode.com link)
- ds9.js - Javascript/Makecode version of game (https://makecode.com/_KhmDoYXCpVdh)
- ds9.py - CircuitPython version (rename to code.py)
- bach.py - helper code for music and sounds for ds9.py
It is a pretty simple game using the Circuit Playground circle of neopixels.
The premise is the space station Deep Space Nine (a blue neopixel) is in motion near the Bajoran Wormhole (a neopixel of shifting color). As the station shifts back and forth, the wormhole remains the same distance (five neopixels away). The station is launching automated probes to the wormhole - A sends them counter-clockwise, B sends them in a clockwise direction. You have ten probes to launch - if they successfully enter the wormhole, there will be a rainbow of neopixels across all ten. When you have sent all ten, you'll see pixels representing how many you managed to send into the wormhole (0-10). For the Makecode version, shake the CPX to restart. For the CircuitPython version press A or B.
Both versions make a sound when launching the probe, and will play a scale or musical flourish when you succeed. To turn that off, slide the switch to the left. To the right turns the game sounds on.
I found the differences in coding Makecode and CircuitPython versions interesting. With Makecode I could have multiple "forever" loops handling different actions - toggling Booleans to activate/deactivate them. With CircuitPython I used a single game loop and controlled the movement of the probe with a Boolean and a counter (it can only go 4 steps). The same Boolean prevents the A/B buttons from launching more than one probe at a time.
Give it a try - see how many you can get to land in the wormhole!
-
Create a Looping Apple Shortcut for Sending Data to itsaSnap Recently I've been experimenting with Apple Shortcuts to interface with the new itsaSnap app. This app lets you interface with Adafruit IO feeds on your phone. With Apple Shortcuts, you can get data from your phone to Adafruit IO. You can, for example, send health data, weather data, even encoded photos.
One topic that comes up in Apple Shortcuts is not being able to easily create an automated Shortcut that loops. For example, having data be sent every 30 minutes. There is a time automation, but you have to setup an automation instance for every time you need the Shortcut to run.
I wanted to figure out a way to do this and found a helpful post on the Apple Shortcuts subreddit that describes using alarms as a workaround. I normally avoid Reddit but that particular subreddit has had very helpful posts with folks sharing their Shortcuts and tips for folks to accomplish what they're looking for.
Flow Chart
I've adapted the suggested Shortcut from the subreddit post a bit. I've found that wrapping my head around the logic can be a little tricky, so here is a visual explainer before we get into building out the Shortcuts.
-
Feather TFT Clock with Gamepad Input This clock project uses USB gamepad input to control its time setting menu. The display uses TileGrid sprites that I made in Krita. The code demonstrates how to use timers and a state machine to build an event loop with gamepad input, I2C real time clock IO, and display updates.
Overview and Context
This clock is a step along the way on my quest towards learning how to build little games and apps in CircuitPython. The look for the display theme is about digital watches and alarm clocks from the 80's and 90's.
Some of the technical bits and pieces from this project that you might be able to reuse in your own projects include:
Menu system for manually setting time and date
USB gamepad input system with edge-triggered button press events and repeating timer-triggered button hold events
Data-watch style display theme with three display areas: 20 ASCII characters at the top, an eight digit 7-segment clock display in the middle, and another 20 ASCII character display at the bottom
Main event loop with gamepad button polling, real time clock polling, state machine updates and display updates
-
Prime Time Python! I think prime numbers are like life. They are very logical but you could never work out the rules, even if you spent all your time thinking about them - Mark Haddon, The Curious Incident of the Dog in the Night-Time
At some point, I realize I consider the Circuit Playground kind of a multi-tool - sensors, inputs, sound output, control of motors and more... actually, maybe more like Doctor Who's Sonic Screwdriver....
This week I worked to reconfigure my CPX to search for prime numbers because... why not? With my memory of the Sieve of Eratosthenes it took no time to find Python code to adapt for CircuitPython. In fact the code pretty much ran fine from the start.
First though, I made a version for Makecode - essentially using the Python code as a model. And then I made the CircuitPython version - actually two versions. More on that later. (And, yes, there is a NeoTrinkey version as well).
It is all in this repository : Eratosthenes
- ESieve.js - MakeCode/Javascript for finding all primes < 1000 https://makecode.com/_H5qFx46rYF1c for Makecode version
- ESieve.py - CircuitPython for finding all primes < 1000 - copy to code.py
- ESievePlus.py - CircuitPython for finding all primes < 8000 - copy to code.py
All three of the above will flash colored lights while searching for the prime numbers. When done, A will pick a random prime and display it, first in binary, then digit-by-digit in decimal version. Pressing B will step through all of the primes it found, displaying in binary. Touch A1 to display the last random prime it found in the random selection from pressing A.
The coding of binary values uses the ten neopixels - Green for 1's and Blue for 0's
NeoPixel: 0 1 2 3 4 5 6 7 8 9
Value: 1 2 4 8 16 32 64 128 256 512
The digit-by-digit display of a number lights up yellow pixels to show a value, with the rest of the pixels blue (so for 0 they all are blue).
- neosieve.py - neotrinkey version (copy to code.py)
- ncount.py - support file for neosieve.py
NeoTrinkey version. It finds all primes < 1000. First it flashes colored lights while searching, then, when done, touching pad #1 will display a random prime digit by digit with binary coding. Touching pad #2 will redisplay the last random prime found.
The "digits" displayed use the binary coding I had in the RRPN Calculator project:
All of the Circuit Python versions (neotrinkey or Circuit Playground), if connected to Mu or a similar IDE, will print information to the REPL.
Code based on https://www.geeksforgeeks.org/python-program-for-sieve-of-eratosthenes/ - MakeCode version written from scratch recreating the algorithm followed in that sample Python code.
Challenges
It seemed ... paltry to ONLY do the primes < 1000 but creating a Boolean array of more than 1000 ran into memory limitations. To fix that, in ESievePlus.py, instead of an array of Booleans, I created an array of 1000 8 bit numbers, initially set to 255 (11111111 in binary) and wrote functions to clear bits (indicating NOT prime) and another to test whether individual bits were 1 (prime) or 0 (not prime).
This introduced another problem - numbers greater than 1023 need more than 10 bits to display - so I modified the showbin() routine to shift all the pixels when displaying the higher bits.
Note: Since it takes a while to display hundreds of prime numbers when you push the B button (over a thousand for the ESievePlus.py version!) - the A button is an easy way to sample the primes that were found.
NeoTrinkey Binary Values -
Work in Progress: Feather TFT Clock This is an update on my current work in progress. I'm making a clock that will use gamepad buttons to set the time and date. The look is about 80's and 90's LED alarm clocks and digital watches. My larger goal is to explore making USB gamepad controlled GUIs in CircuitPython.
For now, I'm deciding how I want time and date setting to work, including how the final sprites will look. To watch my video progress update, click through to the full post.
Video Progress Update
-
Orrery: Put a solar system in your pocket! For hundreds of years, the clockwork orrery has been a way to demonstrate the movement of planets around the sun - as Wikipedia describes them: "An orrery is a mechanical model of the Solar System that illustrates or predicts the relative positions and motions of the planets and moons, usually according to the heliocentric model. "
It occurred to me that, the circular display of ten neopixels on the Circuit Playground might be a way to make a different kind of orrery... so I did. My programs are in this repository and consist of three programs:
-
orrery.js - Javascript/Makecode version for the Circuit Playground (https://makecode.com/_EHeh61h4Dcvo for the Makecode IDE version)
-
orrery.py - Circuit Python version for the Circuit Playground (copy to code.py on the device)
-
neo-orrery.py - Circuit Python version for the NeoTrinkey - again, copy to code.py on the device (you didn't think I'd leave the NeoTrinkey out did you?)
I opted to just do Mercury, Venus, Earth and Mars - adding more planets was too cluttered, plus the relative speeds of the inner planets are easier to see.
All versions start by showing Mercury (pale white), Venus (yellow), Earth (green) and Mars (red) as neo pixels moving at their relative speeds around the sun - from Mercury the fastest to Mars the slowest.
Each can switch to a random setting, changing the color and speeds of the four planets.
Makecode version: "A" stops/starts motion, "B" sets up a random solar system, and "Shake" resets to defaults.
Circuit Python Playground version: "B" sets up a random solar system and "A" resets to defaults
Circuit Python NeoTrinkey version: Touch pad #2 to get a random solar system and pad #1 to reset to defaults
NOTE:
The mechanics of the simulation are simple. Each program has a loop that increments a counter for each "planet." There is a table of periods for each planet, an integer that is 100 times the length of the planet year, so, for example, Mercury's period is 22 and Earth's is 100. When a planet's counter reaches the value of its period, the counter is cleared and the planet's position is advanced one position.
A small orrery showing Earth and the inner planets -
-
LED Pixel Mapping with WLED and LEDLabs Hello, my name is Erin St Blaine and I love making things that light up.
When I'm not writing tutorials for the Adafruit Learning System, I spend my time creating beautiful things, and exploring the meeting of art and technology whenever I get the chance. Over the last several years I've been focusing on creating larger scale LED artwork, home decor and chandeliers. I started out using Arduino with FastLED and learned to code the hard way, but nowadays there are so many software packages out there that I've been learning and exploring them. This article is about my experience of LED mapping my newest chandelier commission using WLED, PixelBlaze and LEDLabs.
What is Pixel Mapping?
Pixel mapping is a technique used to control and program individual LEDs or pixels within a lighting display, allowing each light to be addressed and manipulated independently. By mapping out the exact location of each pixel in a 2D or 3D space, artists and designers can create intricate patterns, animations, and effects that synchronize with the physical layout of the lights. This method is essential for creating dynamic, visually stunning displays, as it enables precise control over color, intensity, and timing across complex setups like LED walls, chandeliers, and sculptures.
A pixel map is needed when the LED layout is not precisely rectangular. Basically, a pixel map forces a non-rectangular shape into a rectangle that can be divided into rows and columns so the animations lay out correctly in the physical space.
My first foray into pixel mapping was making an LED Festival Coat using WLED. This was a fairly straightforward map -- the layout was generally rectangular, with just a few "holes" in the rectangle to account for -- I needed the map to fill in the arm hole areas so that my animations would look even across the whole coat. I used WLED for this, and found it to be a little mind-bending and tricky even with a simple map.
After the success of that project I decided to give mapping my chandelier a try. The chandelier is shaped like a hot-air balloon with eight spokes and no rectangles at all. This looked to be a challenge but I knew the end result could be absolutely stunning if I succeeded.
-
Unboxing a 10 year old Home Theater PC As an avid personal computer (PC) enthusiast, I was quick to adopt the idea of using a computer to serve multimedia content to a large TV for family use over 12 years ago. There were no Apple TV or Mac Mini boxes back then. The idea was to have a PC with multimedia hardware and emulate what a smart TV might provide you now.
Companies had hoped consumers would adopt multimedia PCs for their entertainment centers for awhile. Back in 1998, Gateway introduced the Destination, combining a 27" (tube) television with a Celeron PC for $2000. A higher end unit had a 36" TV with a Pentium II for $4999.
With Windows XP in 2002, Microsoft decided to embrace the multimedia aspects of their operating system. Extensions called the Windows Media Center provided a "10-foot interface" common to today's smart TV users. This worked best with a TV capture card and a computer outputting decent graphics and sound. It had limited success, available for Windows 7 and 8 also, but not popular enough to be kept in Windows 10.
Concepts
Here were ideas for a good multimedia PC of the era (12 to 14 years ago):
- A decent CPU (dual core or better) but one that ran cool so noisy fans could be mimimized.
- A good amount of RAM for Windows Media Center
- A motherboard with several PCI slots
- A mid to middle high end graphics card. Too slow it wouldn't output fast enough, too fast and the heat would require lots of fans. Passively cooled is optimal. Output in HDMI was high end, VGA and DVI was ok.
- A TV capture and tuining card
- A DVD player. A DVD recorder is better, Blu-Ray is a bonus
- Premium sound card
- Fast storage. SSDs were kind of new but one at least for boot and short term storage is great.
- Firewire for video camera capture is a bonus
- Fast networking to a home server for long term storage. Alternatively a fast hard drive.
- An infrared or RF keyboard with integrated trackpad or trackball
- A Windows Media Center remote control
- A front display, LCD or VFD, is a bonus
- SD card reader
- A horizontal cabinet to blend in to the home theater
-
The Highway 12 Band SFX Machine Deadline: We need it tonight
Just eight hours left until tonight's classic rock band rehearsal session and we need some pre-recorded sound effects for three songs. The sound effects (SFX) playback machine design should be easy to use, battery-powered, providing a stereo output for the band's PA system. Sound fidelity is important, but since the sound effect recordings aren't "musical" per se, there's some bit-rate wiggle room to help with storing the sound files.
The band retired six years ago. Because it didn't look like there would be a reunion tour, the previous sound effects machine (the FXM-8 shown above) was stripped for parts and repurposed. The old unit used a dedicated SFX board (Adafruit #2220) with .ogg sound files stored on-board -- something that worked and sounded good, but it wasn't easy to update the stored files. The simple and obvious FXM-8 tactile user interface was also pretty nice in retrospect.
And of course the band's plans changed. We were asked to come out of retirement and play one more gig.
We're going to need a new SFX machine.
-
Using a Motorola Atrix Dock with a Raspberry Pi Computer 2024 Version Rock an Old Atrix Screen/Keyboard/Trackpad Dock Like It's 2012
Back in 2012, folks were getting their first Raspberry Pi single board computers. They initially used a monitor or television, a USB keyboard and a USB mouse, most often items they might of had around the house. This worked fine but it was far from portable. Folks wanted something a bit more laptop-like to develop on the go.
Enter surplus Motorola gear. Motorola made the Atrix line of cell/mobile phones they marketed as dual use as portable phone and computer. To make it a computer, you used a dock which looked like a laptop but had no processor, only USB and HDMI connections. Such docks were being sold wholesale at $60 each.
Folks used a combination of Far Eastern cables and adapters to connect the micro connections on the dock to the full size connectors on the Pi. As you can see below, two adapters and three cables were used. Some guides talked about splicing cables. Here is one guide from Instructables.
The setup worked faily well. Most often the adapters to the Atrix were too big, so some filing of the plastic was needed to get the cables to go on without interferring with each other. It was a slick setup but it could be fragile.
Over time, most folks moved on from using this solution as the Atrix dock supply dried up and new Pi models came along.
The 2024 Atrix Solution
I got my Atrix Dock out to use with a Pi 5 for Adafruit's Show and Tell. Things had changed since the Pi 1 when now using a Pi 5. The one full size HDMI connector is now a pairt of microHDMI connectors. There are more USB ports on the Pi 5.
I wanted a solution with no adapters and no trimming of the plug housings. I went on to Amazon (US) and through brute force (Amazon search is not the most robust) found the following cables:
-
Air Quality Monitor for the Feather RP2040 Remixing the guide from the Ruiz brothers: https://learn.adafruit.com/aqi-case/overview and retrofitting it for the Feather RP2040 & the Adafruit FeatherWing OLED - 128x32 OLED Add-on For Feather
The OLED is quite limited in colors & the Feather RP2040 has a single LED available. Given these constraints this is what I was able to achieve.
All the text on the third row are my own interpretations of the values of each of the categories. Here's an example of how I assigned a text value to the data around the PM2.5 data.
def rate_pm25(pm25_data): if pm25_data <= 12: pm25_color = green pm25_extra = "OK" elif pm25_data <= 35: pm25_color = yellow pm25_extra = "MID" else: pm25_color = red pm25_extra = "BAD" return pm25_color, pm25_extra
-
Sparkle Specs firmware for Adafruit LED Glasses driver Do you have a pair of Adafruit LED Glasses? Have you noticed there is a ton of cool examples and starter projects out there for them? Have you ever wished you didn't have to juggle installing different projects in order to use each one? Do you want to use a Wii Nunchuck for wireless game-like controls? Would you like to also be able to use the Adafruit Bluefruit Connect app to do things like change colors or other settings? Did you ever wish those settings would be saved, even when you turn the glasses off? Do you wish you could install it by simply dragging and dropping a file?
Motivation/Goals
I wrote a bare-bones demo a few years ago using the Wii Nunchuck to control an adaptation of the classic Adafruit roboface project, played with it for a few minutes, and then promptly forgot about it. I recently rememberedI had the glasses kit, and with Halloween around the corner I thought it would be fun to wear them this year, sporting a more robust firmware than my original demo.
I wanted to have a small suite of different modes available to use, and be able to control them wirelessly. The first part of the project was building a small adapter I could use to allow my Nunchuck to pair and communicate with my glasses using Bluetooth Low Energy. With that complete, it was time to write the firmware for the glasses. I wanted the firmware to have the following features:
- It should have the ability to pair with the BLE nunchuck adapter and use the nunchuck for wireless control
- It should have the ability to connect to the Adafruit Bluefruit Connect app to change colors and other settings
- The glasses should still work well even without some form of wireless control
- It should have modes that take advantage of the built-in accelerometer and microphone on the glasses driver board
- It should have an application/firmware architecture that makes it simple to add new modes in the future.
- Optionally, it should have the ability to connect an EEPROM breakout to the remaining Stemma QT port on the other side of the glasses to save changed settings, even when the glasses are powered off.