Getting Started
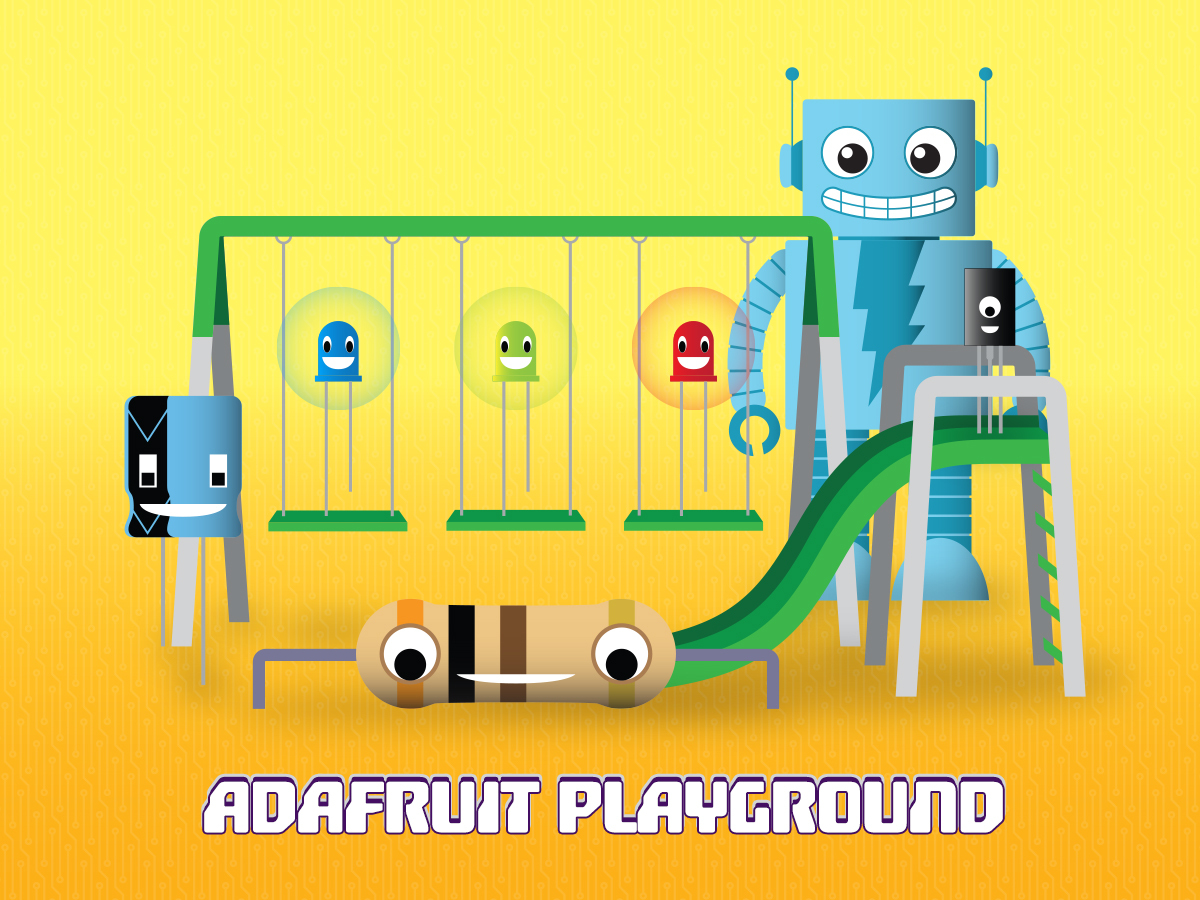
Adafruit Playground is a wonderful and safe place to share your interests with Adafruit's vibrant community of makers and doers. Have a cool project you are working on? Have a bit of code that you think others will find useful? Want to show off your electronics workbench? You have come to the right place.
The goal of Adafruit Playground is to make it as simple as possible to share your work. On the Adafruit Playground users can create Notes. A note is a single-page space where you can document your topic using Adafruit's easy-to-use editor. Notes are like Guides on the Adafruit Learning System but guides are high-fidelity content curated and maintained by Adafuit. Notes are whatever you want them to be. Have fun and be kind.
Click here to learn more about Adafruit Playground and how to get started.
-
No-Code "EnSmartening" an IKEA FÖRNUFTIG Air Purifier - V1 Reuse existing PCB+Dial This project was a personal desire to put Adafruit IO through it's paces, and see how far I could go in recreating a project designed for ESPHome + Home Assistant. Traditionally I would have assumed it was too complex for the Actions and Dashboard of Adafruit IO if I used a Wippersnapper device, instead relying on Node-RED to string things together, but now that I work on the WipperSnapper project I was curious how far it had come / could go.
The basic idea was to take a £60 "dumb" air purifier with a 3-speed dial (£70 with carbon filter) and add a WiFi micro-controller, so that it can be switched on and the speed controlled according to the values sent from a separate Air Quality sensor (Particulate Matter <2.5µm), and a user-adjustable control panel hosted as an Adafruit IO Dashboard.
Is this another silly idea / tribute to insanity, by needlessly bolting the internet and a subscription service to a device, which usually degrades performance and adds built-in obsolescence (not to mention a 22second boot-time)?
Well maybe, or maybe it's just a way of providing people with alternatives, but either way it involves Adafruit IO as an alternative to Home-Assistant / Node-RED or the official IKEA smart-hub + smart devices, and Adafruit's open-source IOT firmware called WipperSnapper as an alternative to ESPHome.
It's possible to use on the free-plan on Adafruit IO (you do NOT need IO+), or offline with a little coding, so I don't feel bad about suggesting it...
Here's a demo video of it in action: (Adafruit IO Dashboard on left, WipperSnapper device page on right, Speed dial of Air Purifier + Wind Sock on Picture-in-Picture video)
-
WipperSnapper Plant Grow Light My mom has two Christmas Cactus plants that have been doing really well for the past few months. However, with winter arriving she had been struggling to find a spot where they were getting enough light. I offered to build her a grow light using DotStar LEDs since they have a color temperature of 6000K, which these particular plants respond to. I decided to use WipperSnapper so that the lights could turn on and off on a schedule and it would be easy to troubleshoot remotely.
I designed up a wooden box in Fusion360. It was designed to be made with 1/2" plywood.
-
Raspberry Pi Stats Display The 3D printed insert can be found here: https://www.printables.com/model/710601-pi-5-official-case-active-cooler-insert-small-came
To get the display to show stats, first install blinka by following the guide here: https://learn.adafruit.com/circuitpython-on-raspberrypi-linux/installing-circuitpython-on-raspberry-pi
Assuming you created a python virtual environment in /home/pi/env path, run the following commands:
cd ~
source ./env/bin/activate
pip install adafruit-circuitpython-ssd1306
Now create your python file called stats_display.py in the /home/pi directory.
Please try running the command:
vcgencmd measure_clock arm
If your output does not start with frequency(0)= then you will need to change the line:
Freq = Freq.replace('frequency(0)=', '')
to match the start of your output in the code file above.
Now try to run the code by calling:
python ./stats_display.py
If the display is working, we can now run this as a service at start up.
-
RP2040 CW Keyer Adafruit Feather RP2040
Noel J Petit, WB0VGI
This project expands on a keyer developed in 2020 using a previous microcontroller and touch sensitive sensors. Keyers should have:
Touch pads for dash and dot
Push buttons for preprogrammed messages (CQ, RST, etc)
Rotary encoders to adjust keying rate
The Adafruit Feather RP2040 has interfaces and a library of code for all three of the above. The advantage of the Feather RP2040 controller over previous microcontrollers is that the 2040 has touch sensitive pins on the controller itself. This means that minimal external devices are needed as most of the control is provided by software. Touch pins were programmed to control dot and dash. Touch control lines need to sense detection over a given time and not sense multiple detection as the key is touched and removed. This “debouncing” is difficult to do in hardware, but the language we use, CircuitPython provides deboucing for touch panels and switches.
In early 2021, the Raspberry Pi Foundation introduced the RP2040 microcontroller chip. They produced a microcontroller board and Adafruit expanded on that board with a Feather Board. The Feather is specifically made to interface with external devices. Adafruit immediately expanded its library of CircuitPython functions for the Raspberry Pi Pico RP 2040. As a microcontroller, the RP 2040 is not meant to have a fully functional operating system with files and interfaces to things like keyboards and mice. The microcontroller is designed to run one program and interface with many different kinds of inputs and outputs (digital, analog, I2C, SPI, USB…).
Keyers provide services to CW operators for an easier life. Most modern transceivers include an internal electronic keyer that uses either the straight key or dual paddle key to key the transmitter. Timing of the dots and dashes depends on the operator (for the straight key) or the radio's internal keyer (for the paddles). In addition to character timing, some radios include the ability to store messages such as 'CQ CQ CQ DE WB0VGI'. My particular Yaesu FT-897’s include dual paddle dots and dashes timing but cannot store messages. Additionally, to change the keying speed, the Yaesu must stop sending, choose one of the menus, change the speed and then continue the CW conversation. I found this challenging and slow. Programmable keyers allow you to change CW speed while sending and to send preset messages with a touch of a button.
To perform these tasks, a microcontroller is needed. The computer is the Raspberry Pi Foundation’s Feather RP2040 -- a small computer built around a dual-core Arm Cortex-M0+ processor with 264KB internal RAM and support for up to 16MB of off-chip Flash. This is a 32-bit computer running at about 133 Mhz. This small computer is built to run small devices with a language called Circuit-Python (CP). CP is a subset of the python language to talk to and display many digital and analog data sources. Included on the RP2040 are pins that can be digital inputs or outputs (high or low voltage), some analog pins (converted to or from binary numbers).
Figure 1
The RP2040 Keyer uses these touch sensitive pads as inputs to key the transmitter with dots and dashes. An additional rotary encoder increases or decreases the CW sending speed. This means I can adjust the speed without having to stop conversing For touch sensors, all that is needed is a small piece of copper coated PC board glued to a metal plate (in this case another PC board) When touched, the output is a high logic value.
Figure 2
The Feather RP2040 Keyer is built in an aluminum Bud Box with the Feather computer on a small breadboard (this allows for changes as needed). The key sticks out the middle on a piece of copper two-sided circuit board soldered to a base piece of circuit board that fits in the top of the Bud Box. One circuit board for the base is 4” x 2 ¾” and bolted to the top of the Bud Box. The key extension is 4 ½” x 2” and soldered perpendicular to the base PC board. The key extension must be notched to fit the edge of the bud box. External capacitive touch pads are wired to the RP2040 with 28 gauge wire and message/speed selection via push button switches atop the Bud Box (see figure 2 and 3).
Capacitive touch keying takes some time to get used to. For the traditional paddle key, the paddles move back and forth and you feel the motion toward the dot or dash. For capacitive touch you must touch the surface to activate the key and remove you finger from the touch pad to stop the dot or dash. You do not get the sensation of moving the key and you must completely be off the touch pad to turn it off. I am still getting used to it. Capacitive touch pads in figure 2 are used as the dot and dash keys. Note that the Bud Box is not heavy enough to be a comfortable key. My MFJ dual paddle key weighs 2.5 pounds, but the Bud box and its circuitry only weighs about 10 ounces. The solution is use heavy washers or other weights in the removable U shaped bottom of the keyer. I have the box up to about 1.5 pounds and it is quite comfortable.
Figure 3
Your laptop/desktop programs the Feather with a simple editor called MU. The MU editor can, edit python files, check python files for correctness, load or store files onto the Feather , and monitor the output from the Feather. Normally the output appears as either interface pins (digitally on or off) or output is the result of program steps such as the python print() statement. Only one program runs on the Feather and it is called "code.py" but it can have many subprograms called from code.py. Once loaded onto the Feather, "code.py" runs and the results are seen on the serial screen of MU. Since the memory on the Feather is non-volatile flash, as long as the Feather has power it will run code.py but without power, the Feather remembers the program and restarts code.py upon power up. Figure 4 is the layout of the inside of the keyer. Beneath the power connector on the left is the Feather and its breadboard. To the right are two 1/8” sockets for keying the transmitters. A toggle switch between them selects the radio to be keyed.
Figure 4
The schematic is simple. The Feather receives power from the USB-C connector or an external supply of 5 volts. I chose both to use up some of the plug-in supplies in my collection. 220 uf and 0.01 uf capacitors filter the supply’s input. RF will get into the 5 Volt DC input so you may need ferrites or repositioning of the wires to prevent the Feather from chaos. Inputs are pins A2-A3 for the rotary encoder, D25 and D9 for the pre-programmed messages, D5 and SCL for the Dash and Dot. The output pin D11 lights an LED on key. Output pin D12 is goes low to key the transmitter. The circuit is wired with wire wrap 28 gauge wire. Cut pins from wire wrap sockets to use as wire wrap terminals on the breadboard. The SPDT switch on the output determines which radio is keyed. This avoids changing the mode of the radio when satellite up and down links are reversed.
Figure 5
A Feather starts life as a simple microcontroller that expects and executable file of type .UF2 as its operating system. You can download .UF2 files from the Adafruit site and see that the device works correctly. When plugged into a laptop/desktop the Feather appears as one of the disk drives on your PC. To do your own programming, load the latest version of Circuit-Python onto the Feather with just a drag and drop. Once loaded, the Feather expects a python program named code.py. The MU editor will load and store programs for the Feather from then on. All the details are available on the Adafruit learning pages.
Additionally, the Feather has many libraries for multiple devices. These libraries are available from Adafruit as a bundle of about 300 files. You will need some libraries listed in the “import’ statements at the beginning of the Python Code. Also, libraries from Adafruit provide interface to all sorts of things: temperature, magnetic field, displays, humidity, servo motors, LED’s, acceleration (which way is up?) and so on. Download the library .zip file and extract all of the libraries into a directory on your laptop/desktop. When you need a library, drag it from your laptop/desktop’s library to the “lib” folder on the Feather.
Before coding the keyer, you can program and test the Feather without connecting the Feather to any peripherals (how's that for cool?). Connect your laptop/desktop computer to the Feather through a USB to USB C adapter and watch the Feather light up. Follow the instructions for set up of the Feather at the Adafruit.com learning pages. This puts in Circuit-Python, downloads and starts the MU editor for you. Get sample python programs from Adafruit's pages as well. Sample code for the Feather Touch and Digital keyers are at links below. Be sure to change the names of the files to code.py to run them on the Feather . I keep the names descriptive on my PC but change them to code.py on the Feather.
A rotary encoder changes the CW speed up and down. The other two push buttons on top of the keyer provide preprogrammed messages. In my case, one is CQ and the other a standard 599 response. The dash and dot keys are inactive during the message.
Once programmed, the Feather can run with just a power supply connected on the Feather side of the box. It automatically starts the code.py program when powered up. 1/8" coaxial connectors on the keyer side connect to the key inputs of the radios.
The parts list has specific Digikey and Adafruit numbers. Everything except U1 – the Feather RP2040 can be substituted for whatever you have in the shop. Resistors, capacitors, and diodes of anywhere near these specifications will work just fine. Capacitive touch pads are made from cut pieces of copper printed circuit board. I found that smaller touch pads work best. The Dot and Dash pads are 5/8” x 3/8” to be very responsive. Keep the pads small as their percentage change in capacitance is more than a large pad when touched. This may require some experimentation.
References:
Adafruit.com Learning Page: https://learn.adafruit.com/adafruit-qt-py
Adafruit.com Products: https://www.adafruit.com/
Sample Program for RP2040 Touch: https://bit.ly/37aWhse
Sample Program for RP2040 Digital: https://bit.ly/373RZTp
Parts List
Chassis Bud CU-3006-A Digikey 377-1093-ND
Printed Circuit Board (10” x 8”) Digikey 182-1017-ND
Mini Breadboard Digikey 1471-1522-ND or Adafruit Product ID 4539
J1-J3 (1/8” jacks) Digikey 889-1821-ND
D1 Diode 1N4004 (any will do) Digikey 1N4004GOS-ND
C1 0.01 uf disc Digikey 1255PH-ND
C2 220 uf @ 6.3 VDC Electrolytic Digikey P829-ND
R1,2 100 ohm ½ watt Digikey 100H-ND
R3,4 10K ohm ½ watt Digikey 1MH-ND
S1,2 Push Button Digikey EG1930-ND
L1 Red LED Digikey L513HD
S1 SPDT Switch Digikey 2368-54-302PC-ND
U1 Feather RP2040 Adafruit Product ID 4884
X1 Rotary Encoder Adafruit Product ID 377
P1 5 VDC USB-C Supply Adafruit Product ID 4298
USB-A to USB-C cable Adafruit Product ID 4472
Figure Captions
Figure 1 – Adafruit Feather RP2040 microcontroller
Figure 2 – Adafruit Feather RP2040 Digital Keyer with push buttons for messages, rotary encoder for CW speed and external capacitive touch sensors for dots and dashes
Figure 3 – Adafruit Feather RP2040 Pin out diagram
Figure 4 – Adafruit Feather RP2040 mounted on Mini Breadboard inside the keyer
Figure 5 – Adafruit Feather RP2040 schematic
-
Building a LEGO Case for Your Projects The first step is to get BrickLink Studio 2.0. This CAD program is intuitive to use, includes almost all bricks available, and features to test your model strength, render 3D visuals of it, view what parts you use and even generate instructions on how to build your model. This is not a guide on how to use Studio 2.0, but it includes a built in tutorial that makes getting started a breeze.
John Park wrote an excellent guide Lego Set Lighting. I used this guide to light up the LEGO Christmas Tree (set #40573) making the candles flicker away. My problem was how do I hide the electronics running everything.
I had the idea to design and build a LEGO model in the shape of a present, but the question was how.
It turns out there is an easily accessible CAD program and method of ordering parts (maybe too easy!).
-
Microchip sample orders! Today I ordered some component samples from Microchip!
Many component companies offer free samples.
Microchip is very friendly for sample orders. Others like TI may request that you buy the parts.
I ordered a ATTINY84A-PU and a MCP2221A from Microchip.
Sample orders are for prototyping and are not to be resold.
In many cases, email domains like gmail.com and yahoo.com are not accepted.
You may get lucky though. :)
If you want, you can attempt to order component samples from Microchip at this link:
(Make sure you have the complete part number.)
https://www.microchipdirect.com/samples
Please don't abuse component samples. -
WaveBuilder: Construct a synthio Wave Table from a List of Oscillators A study to produce a CircuitPython helper library to construct a synthio.waveform wave table object from a list of fundamental and overtone frequencies, amplitudes, and wave shapes (sine, square, triangle, saw, noise).
The WaveBuilder class currently resides in its GitHub repository and can be found in the CircuitPython Community Bundle. The update utility circup can also be used to install cedargrove_wavebuilder.
The objective was simple. Create a realistic approximation of a wind chime sound. Use synthio to stack up a collection of sine wave Note objects starting with the fundamental frequency and mix in a few unique overtones, each with its appropriate volume level. Use the same ADSR envelope for all notes and voilà, we've got a pretty good chime sound. That worked nicely. Let's try to synthesize another voice.
-
Two-hand Mouse Background
I have an older laptop that has a trackpad, but it is not clickable. You have to use the buttons below it. It is quite awkward in general, more so for drag and drop operations. Let's build some alternative mouse buttons to help. With this project you can move the mouse with your usual hand and click with your other hand. This guide covers USB HID Mouse, Debounce, and NeoKeys.
Hardware
- Feather RP2040: A powerful microcontroller board featuring the RP2040 chip. RP2040 Feather
- NeoKey 1x4: A small breakout board with four mechanical key switches, each with a NeoPixel LED. NeoKey 1x4
- USB Cable: To connect the Feather RP2040 to your computer.
- STEMMA QT Cable: To connect the NeoKey 1x4 to the Feather. SEMMA QT Cable
- Key Switches: Many to choose from. You will need 4.
- Key Caps: Your pick. You will need 4.
Setup
- Plug the key switches into the NeoKey 1x4. See NeoKey 1x4 Guide for instructions.
- Connect the NeoKey 1x4 to the RP2040 Feather using the STEMMA QT cable.
- Connect the RP2040 Feather to your computer using the USB cable.
- Install a recent version of CircuitPython: See circuitpython.org for details.
-
Electric Fireplace Teardown and Upgrade with WLED I just moved into a new neighborhood. On my morning walk I discovered the best "neighborhood score" of my whole life: a brand-new electric fireplace, still in the box, with a "free" sign on it, sitting at my neighbor's curb. I snagged it immediately. I've been eyeing these since I saw one at the State Fair a while back. They produce a really cool flame-like effect, and also include a heater module.
Apparently my neighbors were getting rid of it because it "beeps from time to time". Well, being the DIY hacker that I am, I decided to crack it open and see if I could disable the beep, and also upgrade the LED strips inside while I was in there.
Teardown / How it Works
This fireplace has two 12v analog RGB strips -- one at the bottom and one at the top -- plus a custom PCB with super-bright LEDs that control the flames. It comes with an IR remote control and a capacitive touch panel, both of which trigger a VERY LOUD annoying beep whenever a button is pressed. The LED light strips are both programmed to cycle through 14 different color modes, but the lights just change from one solid color to the next with no brightness controls or animations available.
I was really interested to learn how the flame effect was created. Turns out it's a very clever spin on the popular "Pepper's Ghost" illusion. This is the same illusion used at the Haunted Mansion in Disneyland to make ghosts seem to appear in mirrors all around.
-
Jar of Virtual Fireflies We all love the subtle, intermittent glow produced by fireflies in the summertime. Each firefly produces its own unique visual pattern in response to the signals given off by its neighbors. Running through the yard trying to catch them is a quintessential Summer activity for young (and not-so-young) children. Kids will often collect them and put them in a jar to bring in the house to enjoy the light show that the fireflies produce before releasing them to go on their merry, little ways.
This project attempts to replicate the beauty and ambience created by a Mason jar of fireflies. But unlike the "real thing", this virtual jar of fireflies can be enjoyed year-round without having to capture and maintain the little buggers.
Note: This is a cross-post of the project I posted on hackaday.io at: ( https://hackaday.io/project/193890-virtual-jar-of-fireflies ). The majority of the components in the project are from Adafruit, so I thought I'd include it here as well.
The Jar
When I originally designed this project in 2020, I chose to house the virtual fireflies in a plastic Mason jar with a plastic lid. Although it looks more attractive in a glass Mason jar (see pics and vids), I was making it for my son and wanted it to be able to survive being dropped without causing a safety hazard. I built all of the circuitry on a protoboard small enough to fit in the lid of the jar along with the rechargeable LiIon battery. A capacitive touch sensor placed under the center of the lid detects a hand "press" to control turning the jar on and off. A built-in LiIon charger allows the battery to be recharged by connecting a USB charger via a micro-B connector when the lid is off of the jar. Once the jar is powered on, it is configured to operate for 1 hour before automatically powering off (if not manually powered off sooner). This is long enough to allow it to be used as a calming nightlight while children (or adults) are drifting off to sleep.
-
macOS: Built-In Support for USB-Serial Chips macOS provides built-in support for some USB-to-serial converter IC's. Built-in support was added at various times for different chips. In the versions of macOS before support for a particular chip appeared, a manufacturer-supplied driver needs to be installed.
I rolled some old Macs back to their original versions, and then rolled them forward, one version at a time, to test support support for three different families of USB-to-serial chips. I tested by attempting to upload firmware to ESP32 chips using esptool.py.
Summary
FTDI FT232x chips have working built-in support starting in Mojave, macOS 10.14. Specifically, a cable using a FT232RL was tested.
Silicon Labs (Silabs) CP210x chips have working built-in support starting in Catalina, macOS 10.15. Specifically, support for CP2104 was tested on an Adafruit Feather HUZZAH32.
WCH CH9102F chips have working built-in support starting in Ventura, macOS 13. Specifically, an Adafruit Feather ESP32 V2 with the CH9102F was tested.
Tested macOS Versions
Not every combination was tested, since I knew which versions did not have support and did not re-test old versions.
High Sierra - macOS 10.13
Only Python 2 is available in the original installation, so Python 3.11 was manually installed from python.org.
NO - FTDI did not work properly, though it appeared as
/dev/cu.usbserial-AL0157X9
.NO - CP2104 does not appear at all.
NO - CH9102F appears as
/dev/cu.usbmodemNNN
, but does not work properly with esptool.py.Mojave - macOS 10.14
Only Python 2 is available in the original installation, so Python 3.11 was manually installed from python.org.
YES - FTDI with esptool.py works. It appeared as
/dev/cu.usbserial-AL0157X9
.NO - CP2104 did not work, I believe (my notes are incomplete).
NO - CH9102F
Catalina - macOS 10.15
Has Python 3.8.
YES - FTDI works.
YES - CP2104 starts working in this version, appearing as
/devcu.usbserial-NNNNNNNN
.NO - CH9102F does not work properly. Only
esptool.py chip_id
works.Big Sur - macOS 11
Same as Catalina.
Monterey - macOS 12
Python 3.9.6
Same as Catalina.
Ventura - macOS 13
YES - FTDI works.
YES- CP2104 works.
YES - CH9102F starts working in this version. It appears as
/dev/cu.usbserial-NNNNNNNN
. Note the change fromusbmodem
tousbserial
from Montery to Ventura.Sonoma - macOS 14
Same as Ventura. All work.
References
-
IoT Wind Chimes using synthio The Weather Chimes project fills that need. It connects to the Adafruit NTP service for network time and to OpenWeatherMap.org for wind speed data. The wind speed data is retrieved every twenty minutes and is used to adjust wind chime playback in a pseudo random pattern. The chime voice synthesizer is provided by the
CircuitPython_Chimes
class and for this project, is sent to an Adafruit MAX98357A I2S amplifier driving an Adafruit 40mm 4-ohm 3-watt speaker. Although an Unexpected Maker Feather S2 was used for this project, the code should work on just about any ESP32 device that's capable of running CircuitPython.
The Weather Chimes project consists of two primary code files,weather_chimes_code.py
andweather_chimes_wifi.py
. Theweather_chimes_code.py
code is imported via the defaultcode.py
file contained in the Feather S2's root directory. This code contains the primary non-wifi device definitions and the masterwhile...
loop that plays the chimes. It also imports theWeatherChimesWiFi
class fromweather_chimes_wifi.py
.
TheWeatherChimesWiFi
class takes care of all the networking details for connecting and retrieving data from the internet. It also provide helpers for updating and retrieving time and weather as well as properties for including the local time and wind speed. The WiFi class uses thesettings.toml
file for connecting to a home WiFi router as well as parameters needed for Adafruit NTP and the OpenWeatherMap.org API.A fictional
settings.toml
file:A CircuitPython project for indoor "windless" garden chimes that play along with the outdoor wind speed.
-
pixel peace A sign for all times
The neopixels are controlled by the WLED app, to use this you need a WIFI connection. This is a sturdy construction, I have it lashed on my front balcony and it all works fine, it’s winter ready.
Parts and skills needed:
- 1 hula hoop, mine came from the dollar store and is 30 inches in diameter.
- 1 esp32 huzzah / 3405
- 2 1 meter neopixel strip (30 led) / 4801
- 1 5 volt power supply at least 2 amps / 4298
- nylon ties and electricians tape
The tools and skills needed are a glue gun and soldering iron.
The first thing I did was to strengthen the hoop by gluing the insert that was linking the ends with contact cement. Then I attached the vertical strip and huzzah to the hoop as so:
-
Creating a CircuitPython library Bundle for Circup Mad With Power, or just keeping it on the down-low... There's a few good reasons to want to create or use a custom bundle of libraries for circuitpython!
Perhaps you write educational guides and lesson plans and want to have all your custom requirements in once easy to use place. Maybe you've got enough private, rudely-named, or trademark-infringing libraries / drivers / helpers for CircuitPython that you won't add to the community bundle, but still want the convenience of
circup
(the package manager for CircuitPython).I love having a package manager for circuitpython (currently supports USB mass storage based devices, but theres a pull-request for web-workflow suppport).
circup install --auto --py
and I get all my dependencies in readable form, modifiable even if I so wish!So a recent pull request caught my attention, for circup-depenencies that weren't in the pypi repository (python package hosting used by `pip`) yet or at all. That's the exact state of my libraries!
I also was aware of seeing a bundle-add option for circup, used to add custom library bundles, so this weekend was time to bring it all together.
Minor Yak Shaving - Get Library Ready
I've cleaned up the library enough to have a releasable thing, but never sorted the docs. The project is a fork of a Sensirion python library, for which I actually have permission to use their name, but to avoid any issues I wanted to have a way without friction, like a custom library bundle. Anyway the docs use Sphinx, and the read-the-docs theme, but are an old form of config etc, so I cleaned up that to the point of publishing to github pages.
Then I could setup a pyproject.toml file with a
[circup]
section, and inside a field calledcircup_dependencies
with an array of strings. Similar to this:After looking at the current community bundle (which has a build script, and publishes json+zips with each release) I noticed the dependencies were only setup for a requirements.txt and not the new pyproject.toml circup_dependencies section. The build script did however include the requirements.txt and pyproject.toml for each included library in the built release assets (a zip for each mpy/circuitpython version plus a python file version).
As a result of this I've included fake requirements in my requirements.txt (they will actually pass and install with python on a pc as the names match the official libraries. Then the two circuitpython dependencies are included in pyproject.toml as described above.
The last thing was that there were two libraries for release, the Sensirion SEN5x device driver, and a base Sensirion I2C python driver. Both of these need to be submoduled into my bundle repo, in a similar fashion to the official community bundle repo.
Create the bundle
Simplest for me was to fork the community bundle, then clone it locally and
git submodule deinit --all -f
and delete all the submodules, then add the two of my own, and tweak the readme. I also stopped the CI script from attempting to publish to AWS S3. It's worth noting it will error if the submodules commit reference doesn't match latest tag.The usual
git submodule add
, for each library to be included, and if you update things in the driver repos then craft a new release for them and then come back to the bundle repo and enter the library submodule folder andgit pull
then commit and push those changes, then create a new release of the bundle. Probably equally wise to use the update script or tweak the CI script to update and release (could be scheduled).Use the Bundle
circup bundle-add
<github_owner>/
<github_repository_name>e.g. for https://github.com/good-enough-technology/CircuitPython_GoodEnough_Bundle
circup bundle-add good-enough-technology/circuitpython_goodenough_bundle
then install newly available package:
circup install sensirion_i2c_sen5x
(I prefer to add the --py option to get readable python instead of .mpy module files)
-
CircuitPython Helpers Project Maybe It's Just Me
As I work on different projects I find myself implementing similar things across projects. This has led to a lot of copy/paste work. Which in turn leads to having to update multiple projects when I find an issue or improvement.
So I decided to start a helpers project. It is essentially a set of wrapper classes that I can use across projects.
What's In The Big Pink Box?
I've just started to build this project and have started with the ones that were in use with my current projects. I plan to add more as I move to other projects.
- time_lord.py
- Provides a time singleton that supports RTC if desired
- Currently does not support using SPI and Network to get the time (maybe in a later release)
- local_logger.py
- Provides a logging singleton that supports adding/removing different handlers and accessing files on your SD card
- rotating log files is currently incomplete
- local_mqtt.py
- Provides an MQTT singleton that uses SSL by default
- Supports publishing independently or via the MQTTHandler in adafruit_logging
Any Gotchas?
I have done my best to keep all the helpers independent. I didn't want to force anyone to use local_logger just to use the time_lord wrapper.
The responsibility use the helpers together falls to the program.
How Does it Work?
I clone this project into the project in which I want to use the wrappers. From there I just copy the wrappers I'm using to my microcontroller.
Example code - implementing time_lord.py without using a real-time clock:
...
import socketpool
import wifi
import time_lord
...
pool = socketpool.SocketPool(wifi.radio)
my_time = time_lord.configure_time(pool)
...Example code - implementing local_logger with time_lord and a real-time clock:
...
import rtc
import socketpool
import wifi
import time_lord
import local_logger
...
pool = socketpool.SocketPool(wifi.radio)
rtc = RTC() # using a QTPY ESP32 Pico
my_time = time_lord.configure_time(pool, rtc)
my_log = local_logger.getLocalLogger(use_time=True)
...Example code - implementing local_mqtt with local_logger without time_lord:
...
import local_mqtt
import local_logger
...
my_log = local_logger.getLocalLogger()
my_mqtt = local_mqtt.getMQTT(use_logger=True)Where To Get the Project
You can find the project on Github
Please feel free to contribute!
- time_lord.py