Getting Started
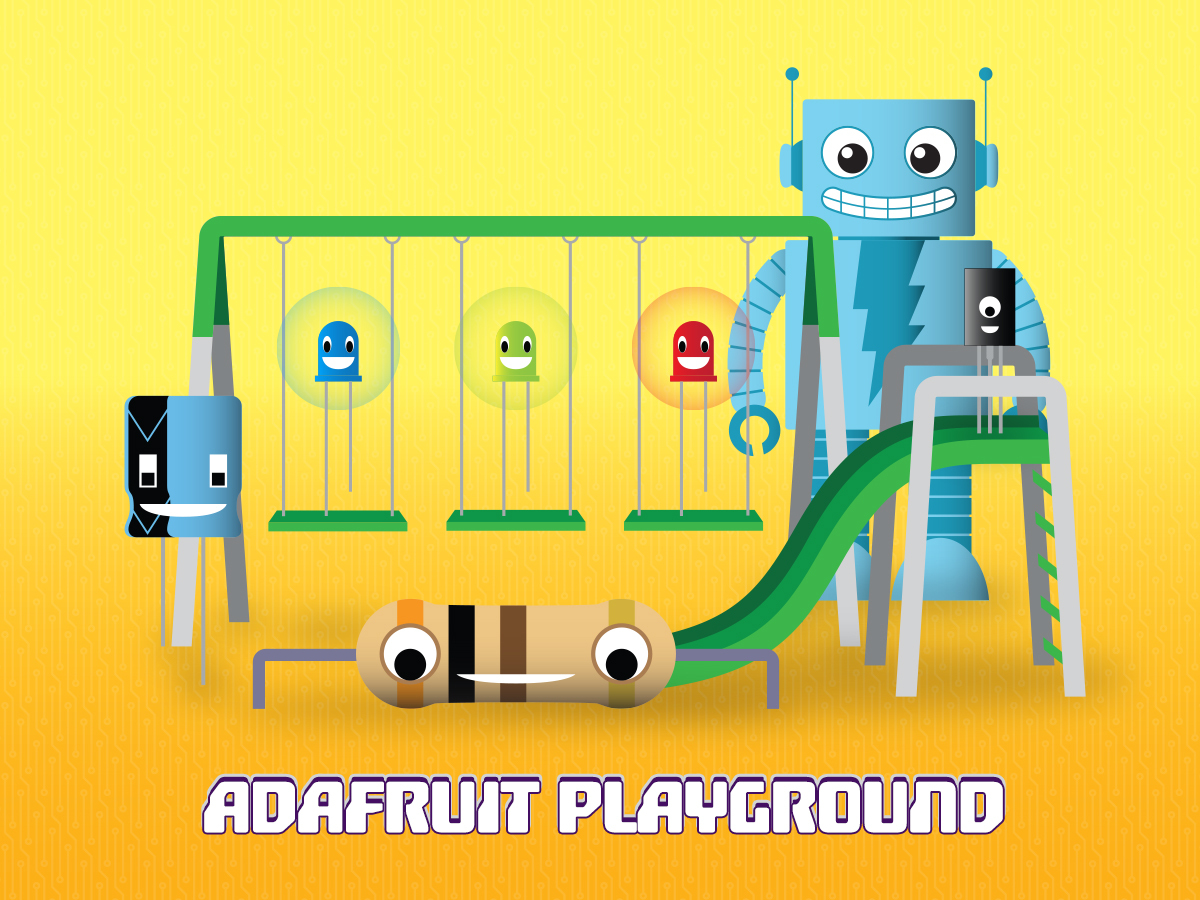
Adafruit Playground is a wonderful and safe place to share your interests with Adafruit's vibrant community of makers and doers. Have a cool project you are working on? Have a bit of code that you think others will find useful? Want to show off your electronics workbench? You have come to the right place.
The goal of Adafruit Playground is to make it as simple as possible to share your work. On the Adafruit Playground users can create Notes. A note is a single-page space where you can document your topic using Adafruit's easy-to-use editor. Notes are like Guides on the Adafruit Learning System but guides are high-fidelity content curated and maintained by Adafuit. Notes are whatever you want them to be. Have fun and be kind.
Click here to learn more about Adafruit Playground and how to get started.
-
Failing with CircuitPython How to fail with CircuitPython
All code will have errors. You might find it sooner or later, or the error might be in your code, in a library that you are using or even in CircuitPython itself!
It is also very common to write some code on your lab environment, test it a bunch of times, then take it out into the field and watch it fail in lots of ways you would never though of. Exactly the same happens when you give your device to a user or a kid who will test it in ways that will make your coding skills feel silly.
In my case this is happening for a weather station. This should be a simple project, right? Grab some data from a sensor like a Stemma QT thermometer, then send that data to Adafruit.IO and watch it get graphed in a lovely dashboard. Or at least that was my expectation for this project.
As you can see, I started getting missing data for days, and only when I went to the semi-remote location where I am testing my weather station, and manually rebooted the devices, I would get start getting data again. But now the graph is ruined, it has a large gap in the data and to all whom I showed the graphs, the first thing they mentioned is 'what happened to the missing data?'. Now what do I do?
Look at the last hours of the graph. Even if I am missing only a little bit of data, it looks terrible right? You, the reader have no idea how hot it is during the day, how cold it is during the night, or what is the coldest hour. Your eyes go straight to the missing data at the end, with the graphs looking like a shark tooth and being jumpy. What a disaster.
I thought about blaming Adafruit.IO or maybe CircuitPython or maybe it's the fault of the ESP32 that is reading the data. Then I thought about adding extra hardware to it like a TPL5110 power timer but that would require some soldering and would break the wifi workflow that I love so much from CircuitPython 8.
I cloud also get my code full of
try
andexcept
Python blocks on all of the parts of my code. To be honest, I did tried to do it this way, but things would still keep failing so I kept adding moretry/except
blocks until the code looked terrible. I was this close to having a project that was not fun for me, and that I would not like to share how it works with this amazing community that makes CircuitPython what it is. Not sharing? That doesn't sound like me at all. Time to do things a different way.Loving to fail
There is a bit of a hidden gem in CircuitPython and it's the
supervisor
module. I was only able to find a little bit of technical documentation without a simple example that I could just copy and paste into my project, and I also found another example which was close to what I wanted to do in Tod's CircuitPython tricks.After staring at the code I had available for a couple of minutes, I realized that this is what I had to do. I needed to let go of my impulse of keep the code failure free, and embrace CircuitPython failing. I would let if fail any time it wanted, and then I would ask it to try again.
With two simple lines, my weather station code can now fail because the wifi router is down, because the Stemma QT cables had a problem with talking to the sensor, because it was running for so long that it was finding errors in the CircuitPython base code or the request library. It doesn't matter, the board will accept that something weird happened, and would try again. Try this in your code and love to fail as much as I do now!
import supervisor
supervisor.set_next_code_file(filename='code.py', reload_on_error=True)
It should not be this simple, right? Here's how it works:
In the first line of code we are calling a library called
supervisor
. Don't worry, you don't have to install anything, it comes as a standard CircuitPython library and will probably work on any board that you have CircuitPython running on. What it does, is talk to one of the internal parts of CircuitPython and modify how it will behave.The second like, is the one that changes how it works. It's using a function called
set_next_code_file
. It's normally used so that you can run the well knowncode.py
file and if something happens, you can define a second file with another name and that code would do something like recover things, blink a light or beep to indicate that it will not behave as it would normally do. I am not calling a second file, instead I'm telling it to go tocode.py
so that it can try again.And my favorite part comes with the code that says
reload_on_error=True
. The way CircuitPython works is that when it fails it will take you to the REPL console, and show you why it failed. This is a good thing as that is the way that we learn, we make mistakes, the REPL shows us what those mistakes are, and we go and fix them.But for my weather station, once the code was ready and it was doing what I wanted, if there is a failure like the wifi is down, it would go to the console and wait for me to do something. This weather station is located on a dry forest in Costa Rica and it doesn't know that is not next to my computer where it's easy for me to tell it to try again. So what
reload_on_error=True
tells it to do, is to change what it would have usually do and take me to the console, and instead it will do something similar as if I was close so the board, pushing the RESET button every time something unexpected happens.This way, CircuitPython will keep on trying over and over until wifi comes back, and hopefully the graphs will have smaller gaps in the data, and they will look much better and should be able to work like a weather station should. Read the thermometer. Send it to Adafruit.IO. Go to sleep to save some battery.
I love you CircuitPython! You and me can fail as many times as we need to, as long as we do it together and without feeling bad. <3.
-
Tell me a story, Neo Trinkey! Once upon a time.....
..... I remember reading a story in "Summer Weekly Reader" (I think by Isaac Asimov) about a robot that told stories to the young boy who owned it. Mostly fairy tales, till one of the boy's friends loaded a tape with more modern info like spaceships (and robots!).
Now, I don't have that robot... but I have a Neo Trinkey and CircuitPython - that's more than enough.
My repository TellMeAStory has five files, CircuitPython code: scifi.py and prt.py, and text data: planets.sf, aliens.sf and ships.sf. Copy those to a Neo Trinkey, renaming scifi.py to code.py. When the program runs, touching "1" chooses a planet name, and touching "2" will generate a short story. In code.py, if REPL=True, you'll need to be in an editor like mu to see the text. IF you change REPL to be False, then it will use HID support to send the text as if typed on a keyboard! For example:
(touching 1)
Broria KBO6
(touching 2)
Black Sparrow
lifts off from
Broria KBO6
with ancient artifacts
and is damaged in an explosion
and tumbles out of control till emergency crews reach them
Suddenly a ship appears from Notania warning of imminent Bhisih attack.
Planets, Aliens, and Ship names were generated on fantasynamegenerators.com - you can edit the lists with your own choices. And you can rewrite the story elements to your own liking!
[Note: updated to work with CircuitPython 9.x. I moved the lists of aliens, ships and planets to external files to avoid memory allocation problems.]
-
Doggy Buttons! Inspiration
This project was born from two desires. One, see if our dog could be taught to use buttons without spending piles of money. Two, to make use of an ancient Raspberry Pi.
Lots of people have done a project like this, and it isn’t necessary to use a Raspberry Pi. That just happened to be the only unused piece of electronics I had on hand with audio output. But, I will say that I definitely recommend this approach for a couple reasons. Firstly, it’s very easy to get running in Python with Adafruit’s Blinka library. Second, it leaves lots of room for growth (both in number of buttons and features like button presses sending digital messages).
Be sure to read all the way to the bottom for a cute puppy photo!Materials Used
-
Raspberry Pi (low cost model A)
- Gifted by a coworker who was going to throw it out
-
16GB (full size) SD card
- Came with my 3D printer, but is overkill for that purpose
-
Audio cable
- From a box of junk computer parts
-
Audio amplifier
- Included in a box of junk that got shipped to me
-
Speakers
- From an old CRT TV
-
Jumper wires
- Reused from multiple projects
-
Keyboard breakout
- Hand made from salvaged diodes, jumper headers, perf-board, wires, and solder. Let's call it 50% recycled.
-
3D printed buttons (and some hotglue)
- Technically new, but I don't count 3D prints if they displace buying other plastic
-
Electronic buttons inside the prints (also a boot button shutdown switch)
- All salvaged from ewaste or otherwise discarded
-
Wooden mounting board
- Had to buy it from a hobby store
Salvage percentage ~83%! Hard to give an exact number... do you measure by count? By volume? By mass? By value? Do you count new tools you had to buy? Whatever, I'm going to call it 83%.
-
Raspberry Pi (low cost model A)
-
Teach your NeoTrinkey Morse code! C3P0 can talk. R2D2 expresses what he's thinking with beeps and boops. What can you do with a Neo Trinkey?
How about.....Morse code? It's simple, and four neopixels is more than enough to say anything! Here's how I set about doing it.
The great thing about Circuit Python is you can just plug in a device and use any editor on the code, but I like to use thonny as my IDE. Thonny gives you easy access to the REPL and you can have plenty of print() statements for debugging.
-
Spock on a Chip! I love using the random library - it's a simple way to introduce variety into simple programs and here's one example, what I call "Spock on a Chip." Here's a link to the repository.
Copy the files to a NeoTrinkey, changing "spock.py" to "code.py" - when it runs, it occasionally blinks lights, but when you touch one of the pads, it will blink and then pick a random quote out of the "spock" file and print it. If REPL=True, you'll need to be in an editor like mu to see the text. IF you change REPL to be False, then it will use HID support to send the text as if typed on a keyboard! Just the way to jazz up your email! Edit the "spock" file to add your own favorite quotes from everyone's favorite Vulcan.
What's going on?
There are two functions that are used to deliver the quotes. len_file(filename),
def file_len(filename):
with open(filename) as f:
for i, _ in enumerate(f):
pass
return i + 1
and wisdom(file_name).
def wisdom(filename):
qs = open(filename)
for i in range(random.randrange(file_len(filename))+1):
quote = qs.readline()
quote = quote.rstrip()
cmpthink()
qs.close()
return (quote.rstrip())
Give a file name, wisdom() calls len_file() to determine how big the file is, then uses random.randrange() to select the text - that means you can add or subtract from the "spock" file without needing to update the program.
The fun thing with this code is, it can be reused for other purposes. I've already used it to put together a "story" program that uses multiple source files (aliens, suns, planets, etc.) that can be drawn from to generate story lines. It could be used for a diceware style passphrase generator. And, of course, it doesn't have to be Spock on a chip - you can fill the "spock" file with any quotes you choose.
Fascinating. Is this logical? -
NeoTrinkey Tool Kit! It's no secret I think the NeoTrinkey is wonderful. It's small, it's cheap and it runs CircuitPython! Who could ask for more??
As I played with it, one thing I found handy was making some helper modules to assist in building programs - three that are particularly handy are:
-
morse.py - translate text to Morse code, also defines touch pads for input
- def docode(text): # display given text in Morse code
- def blinknum(num,color): #count out a number in a color
- def compthink(): #blink out all the colors when computer "thinking"
-
prt.py - provides a function to print text to the REPL *OR* using HID to send it as typed text.
- def prt(text, REPL): #prints text to REPL if REPL=True; otherwise uses HID to send as typed text
-
ncount.py - blinks numbers, or displays 0-15 in binary.
- def docolor(color): #briefly set all pixels to color
- def blinknum(num,color): #blinks num times in color
- def binnum(num,color): #display num%16 in binary on pixels with color
Using The Modules
Here's a very short program that blinks out, in Morse code some Bible verse fragments (in Klingon - my "nickname" is mrklingon, after all):
from morse import *
John316 = "vaD joH'a' vaj loved the qo'"
Isaiah263 = "Duvoqchugh, vaj rojna'Daq Da'av, DuHarmo'"
Psalm231 = "DevwI'wI' ghaH joH'a''e' jIneHbe'."
while True:
docode(John316)
docode(Isaiah263)
docode(Psalm231)
Pretty simple - it just blinks the code over and over. You could plug it into a power source and let it run - and you could choose your own text. Plugged into a computer running Mu, you would see the text being printed over and over as well.
Using the prt.py module, I wrote magicquest.py. This uses morse.py for the touch pad initialization, and the "compthink()" blinking. Touching pad 1 creates a fantasy character (race, class and name), and pad 2 generates a short story about the character's adventure. I used fantasynamegenerators.com to come up with place names and more.
If you change the value of REPL in the code you can decide if the text gets printed in the REPL using Mu or Thonny, or if it is sent as if typed using a computer.
REPL = False #"printed" text from prt() goes as if typed
REPL = True #"printed" text goes to REPL
I used the ncount.py module's binnum() function in two progams, cards.py and orbit.py.
For the cards.py program, the neotrinkey shuffles a deck of cards when you touch pad1, and pad2 draws a card and prints it in the REPL but displays it as a binary number (1-13) and color
suits = ["diamonds","clubs","hearts","spades"]
scolor = [gold,blue,red,green]Touching 1 and 2 together shuffles and draws three cards.
orbit.py blinks a purple NeoPixel around and around at a speed (sort-of) appropriate to the planet the ship is at - from Mercury to Pluto. This uses binnum() to light up the number 1,2,4, or 8 to move the position around and around the four NeoPixels. Touching pad 1 makes all NeoPixels flash gold (closer to the sun) and shifts to the planet that is next closer to the sun. Touching pad 2 makes all NeoPixels flash blue (farther from the sun) and shifts to the planet next farther out.
Pads 1&2 together make jump the position either to Mercury or Pluto depending on whether you are closer than Mars or at Mar or farther out.
The name of the target planet is printed to the REPL and the orbit speed speeds up or slows down depending on which direction you have moved. (Note: the "speed" is just a factor based the position (planet 1-9) divided by ten. It is not really correct for the real planets).
Adafruit Neotrinkey -
morse.py - translate text to Morse code, also defines touch pads for input
-
How about.... a 4 Pixel Video Game?? Here's a project I had fun putting together using my NeoTrinkey toolkit to make a tiny little video game.
I call it "Galaga" - and it uses the ncount.py and morse.py helper modules.
Gameplay:
- An enemy moves back and forth at random in the 0 and 3 neopixels.
- Touch 1, or 2 to "shoot" the moving enemy (green pixel).
- If you are lined up with it, you get a hit. If not it's a miss.
- A hit blinks gold for the count of hits. If you miss, you blink the number of misses in pale blue.
- A win is 5 hits, and losing is when you have 5 misses or run out of missiles.
- Winning is followed by multicolored blinking.
- Following loss/win the number of missiles, score and miss count are reset and game restarts.
- NOTE: connected to Thonny or Mu, you can see "boom!" and "miss" printed when you hit or miss. Plus notification when you win (or lose).
-
Faster SD writes with ESP32 and SDIO Better SD write performance is probably a common goal. My project uses the Adafruit SD Micro SDIO breakout, the Adafruit OV5640 Camera breakout and the Unexpected Maker ESP32S3 feather in a compact stack (with a custom PCB to connect them) that fits in a GoPro waterproof case. I want GoPro-like video recording but with BLE time synchronization and start/stop functions with multiple cameras. It's like a GoPro but it's not, it's a NoPro!
I'm programming with the Arduino IDE and using the SD_MMC library. The ESP32S3 has an SDIO interface with programmable pins, which the library supports. But for the longest time, I could only get sustainable write speeds of 150-200 kilobytes per second. I'm using a Sandisk Extreme V30 32GB which should get 30MB/s. For the frame rates and resolution I want, I need about 3MB/s.
I initially played with the file system buffer size but never got consistent results. I might occasionally get a few frames written at target speeds but never a whole video. So I set my code aside and started from scratch to explore how to get better speed.
There definitely seemed to be a dependence on file buffer size as well as on the size of data being written. So I tried lots of combinations and got the same results - usually about 150-200 kB/s but occasionally over 3MB/s.
But I had noticed a pattern of good performance when the write lengths were certain powers of 2 so I tried multiples of 512 bytes and got much better speeds. With a file buffer size of 4096 and data sizes over 75kB I could get consistent speed over 3MB/s, just what I need. 4096 also gets consistent small writes at over 1MB/s. Buffer sizes of 8192 and greater actually worked worse.
-
RGB Matrix Word Clocks I was looking for a clock that would tell the time in words rather than just hands (analog) or numbers (digital). I found a few for sale on places like Amazon and eBay, but none of those particularly stood out, so I decided to create a customizable one myself.
I designed and built two variants. Both use the same RGB matrix panel. Both use CircuitPython on the ESP32-S3, but one uses the Matrix Portal S3, and the other uses a standalone Feather ESP32-S3 with an Adafruit RGB Matrix Featherwing Kit. The Matrix Portal version requires no soldering; the Feather version requires some simple soldering. I will show both here.
The text is left justified, vertically centered on the display and each line is in a random color which changes every time the minute changes. Since you control the CircuitPython code, you can change this formatting any way you choose, e.g. same color lines, individually horizontally centered lines, etc.
Common Hardware
-
It's Koozie-licious! (3D Printing) If you have a lot of koozies and are looking for a custom way to store and access them, this koozie holder might just be for you.
My niece requested a koozie holder for her home so I designed and printed this for her. I've made it so it's configurable.
The text that you see in the photos is what is in the STL.
What can be configured?
- Height - You may want bigger or smaller!
- Width - current is for what I think of when I hear "koozie"
- Depth - see above
- Text
- Font
- Size
- Depth
- Spacing
- One side print or both
- You can also download the source code and change up anything about it
Details of this print
- 3D Printer: FlashForge Adventurer 4
- Filament: 3D Printlife Recycled PLA, color: Night
- Supports: Yes
- Nozzle temp: 220c
- Print bed temp: 55c
- Raft: No
- Ironing: Yes - This may be specific to the FlashForge slicer but it puts a smoother finish on the piece
- Total print time: ~57 hours
Where to get it
Photo shoot!
-
Space War - A game for the MagTag # Space War - Magtag
This started out as Star Trek in BASIC written by Mike Mayfield in 1972. It was completely text based, designed to run a teletype for input and output. As did any number of computer programmers at the time, I grabbed a copy and changed and enhanced it. Eventually renaming it to Space War so I didn't have to keep all the Star War references. I don't have any of the original listings. There very well may have never been a complete hardcopy listing. A listing at 10 characters per second on roll paper wasn't something you did often.Eventually, I converted a version to Fortran to run on an IBM mainframe on a CRT that had an addressable cursor. A while ago, I did find a listing of that version (data 1977). And I had an extra MagTag that I wanted to try doing something more interactive than display the weather, or motivational quotes. My first thoughts were that it should be pretty easy to port the Fortran over to Python.
I make no claims that this is a 'port', or 'conversion' of that Fortran program. That program was a convoluted mess of GOTOs and GOSUBs and clever Fortran hacks (and a serious lack of comments). About all I actually
saved were the instructions and the program flow.The MagTag is actually much more powerful than that GE265 or the IBM so I wasn't worried about the program size. There are two issues that I had to spend most of my time on.
1) There is no keyboard on the MagTag. Just 4 buttons. So "typing in" a command was out of the question. And if one button is used for "Next" and one for "Cancel" that meant only two left for commands.
2) The second issue was the eInk display. More specifically, the refresh rate is approximately 3 seconds between changes. So you really can't give feedback whena button is pressed. This was a real challenge when the commander needed to enter a four digit coordinate to warp to.
There are addon's that could easily attach to the Magtag to address these. But I wanted to be able to play it on a "stock" Magtag with no additional components.
And I added some comments.
I think it turned out pretty good. Though there are still some things left to implement.
https://github.com/vrtisworks/Space-War---Magtag
-
Desktop Multifunction Device: Clock Here is the first sketch: a simple clock.
This script provides a simple digital clock display on the Feather ESP32-S2 TFT. The time is updated every 0.1 seconds, and the display shows a circle and the current time in a specified font. Built on the Desk of Distress.
Next Steps:
Temperature and Humidity (ADT7410)
-
SPI on D10-13 using Metro RP2040 and 2.8" TFT Touch Shield v2 ( ADA# 1651 ) My current project requires an ST powerSTEP motor driver UNO R3 shield with the Adafruit Metro RP2040 requiring SPI on the normal D10-13 pins. I am also using the AdaFruit 2.8" TFT Touch Shield in the alternate configuration with SPI on D11-13. Getting these to work turned out a little painful, as SPI on D10-13 doesn't work out of the box. I had used the ST powerSTEP motor shield in other Arduino UNO projects, and was caught by surprise when it's D10-13 SPI didn't work with the Metro RP2040.
Required is a simple hardware hack to swap pins D10 and D13 on the UNO R3 connectors. I did this with some extra headers with D10 and D13 broken out and swapped with blue wires to the Metro RP2040 pins on the back of the board.
The Arduino board files for the Metro RP2040 required enabling SPI1 since D10-13 can not be used with SPI0 on the RP2040, and swapping the D10 and D13 pin configuration to match the hardware header hack.
in rp2040/3.6.2/variants/adafruit_metro/pins_arduino.h, set:
// SPI1
#define PIN_SPI1_MISO (12u)
#define PIN_SPI1_MOSI (11u)
#define PIN_SPI1_SCK (10u)
#define PIN_SPI1_SS (13u)#define SPI_HOWMANY (2u)
in rp2040/3.6.2/variants/generic/common.h, change:
static const uint8_t D10 = (13u); // swapped D10 and D13 at pin headers
static const uint8_t D13 = (10u); // swapped D10 and D13 at pin headersUsing TouchPaint to verify the SPI hardware and configuration hacks, required changing the initialization to SPI1.
Adafruit_ILI9341 tft = Adafruit_ILI9341(&SPI1, TFT_DC, TFT_CS)
I had rewritten TouchPaint to add calibration for the touch function, since the software defined touch values worked very poorly with my board. The touch points with a small X and Y didn't pick up, leaving a good sized unusable area in that corner, and along the all the edges. Lowering the touch pressure from 300 to 100 got the full screen area touch working, but there was a several mm mis-registration between the pen contact, and the drawn pixels. I also cleaned up static defines to use the calibration values, returned hardware values, and dynamic pallet size based on an array of pallet colors.
The debug serial prints for calibration need to be moved below the two calibration calls for debugging. i just moved them into a single ifdef.
-
Desktop Multifunction Device: Hardware Would you like to have a desktop clock, weather station, dice roller, timer, etc? This project describes a hardware solution you can use. It is based on a TFT Feather and a Feather Doubler board, with a Temperature and Motion Wing.
Equipment:
- ESP32 Feather with TFT display: There are several to choose from.
-
Temperature Motion Wing which includes:
- ADXL343 triple-axis accelerometer
- ADT7410 precision temperature sensor
- FeatherWing Doubler is the 'frame' of the device.
-
Female Headers for the Doubler:
- Use the black ones included in the pack OR
- Color Headers in your choice of colors.
- Use the black ones included in the pack OR
-
Sound Reactive Neopixel Brass Bell Cover I'm in a radical marching band called Brass Your Heart, and I wanted to make our instruments light up when we play them. I started this project by making one for my friend who plays a marching baritone. Below is a mostly step by step process for how I made them using: sewable neopixels, conductive thread, snaps, a Gemma M0, and an electret microphone.
Step One:
The diameter of the baritone measured 26 inches, so I used tailor's chalk to draw the outer circle you see here. Then I drew another circle, about an inch in for the lights, so that the neopixels and the conductive thread is far away from the metal of the instrument. I also make 8 markings where the neopixels would go and started to sew them into position with regular black thread.